前端开发中的防抖与节流技术详解 | 优化高频事件处理
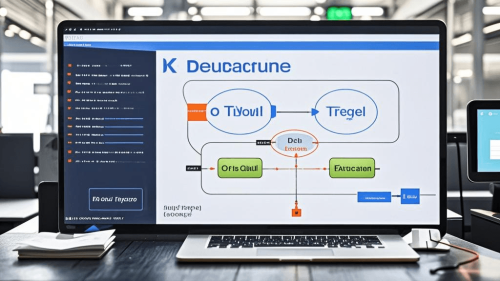
在前端开发中,防抖(Debounce)和节流(Throttle)是两种常用的优化技术,用于控制事件触发的频率,特别是在处理用户输入、窗口调整、滚动等高频事件时非常有用。下面我将详细解释这两种技术,并提供实现代码。
1. 防抖(Debounce)
防抖的核心思想是:在事件被触发后,等待一段时间(延迟)再执行回调函数。如果在这段时间内事件再次被触发,则重新计时。这样可以确保在事件停止触发一段时间后才执行回调,避免频繁触发。
适用场景:
- 搜索框输入时的自动补全
- 窗口大小调整时的布局调整
- 按钮点击防止多次提交
实现代码:
function debounce(func, wait) {
let timeout;
return function(...args) {
const context = this;
clearTimeout(timeout);
timeout = setTimeout(() => func.apply(context, args), wait);
};
}
// 使用示例
const handleResize = debounce(() => {
console.log('Window resized!');
}, 300);
window.addEventListener('resize', handleResize);
2. 节流(Throttle)
节流的核心思想是:在一定时间间隔内,只执行一次回调函数。无论事件触发的频率有多高,回调函数都会按照固定的时间间隔执行。
适用场景:
- 滚动事件的处理
- 鼠标移动事件的处理
- 游戏中的按键处理
实现代码:
function throttle(func, wait) {
let lastTime = 0;
return function(...args) {
const now = Date.now();
if (now - lastTime >= wait) {
func.apply(this, args);
lastTime = now;
}
};
}
// 使用示例
const handleScroll = throttle(() => {
console.log('Scrolling!');
}, 200);
window.addEventListener('scroll', handleScroll);
3. 结合防抖和节流
在某些场景下,可能需要结合防抖和节流的特性。例如,在滚动事件中,可以先使用节流来限制回调的执行频率,然后在滚动停止后再执行一次防抖操作。
实现代码:
function debounceThrottle(func, wait, throttleWait) {
let lastTime = 0;
let timeout;
return function(...args) {
const context = this;
const now = Date.now();
if (now - lastTime >= throttleWait) {
func.apply(context, args);
lastTime = now;
}
clearTimeout(timeout);
timeout = setTimeout(() => {
func.apply(context, args);
lastTime = now;
}, wait);
};
}
// 使用示例
const handleScroll = debounceThrottle(() => {
console.log('Scrolling with debounce and throttle!');
}, 300, 100);
window.addEventListener('scroll', handleScroll);
4. 使用 Lodash 库
如果你不想手动实现防抖和节流,可以使用 Lodash 库提供的 _.debounce
和 _.throttle
方法。
import _ from 'lodash';
// 防抖
const handleResize = _.debounce(() => {
console.log('Window resized!');
}, 300);
window.addEventListener('resize', handleResize);
// 节流
const handleScroll = _.throttle(() => {
console.log('Scrolling!');
}, 200);
window.addEventListener('scroll', handleScroll);
总结
- 防抖:适用于事件触发后需要等待一段时间再执行的场景。
- 节流:适用于需要在一定时间间隔内限制事件触发频率的场景。
根据具体需求选择合适的优化技术,可以有效提升前端应用的性能和用户体验。