前端开发中localStorage数据失效时间设置
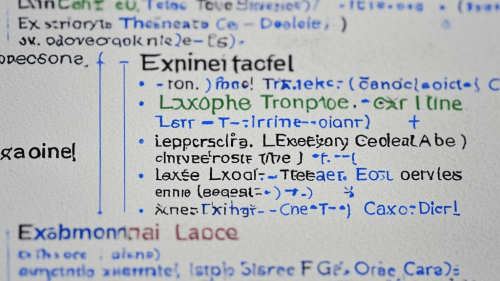
在前端开发中,我们通常使用 localStorage
或 sessionStorage
来存储数据。localStorage
是持久化的,除非手动清除,否则数据会一直存在。而 sessionStorage
的数据在页面会话结束时(即关闭标签页或浏览器)会被清除。
如果你希望在 localStorage
中存储的数据在一定时间后自动失效,可以通过以下方式实现:
1. 存储数据时同时存储时间戳
在存储数据时,同时存储一个时间戳,表示数据的存储时间。
function setLocalStorageWithExpiry(key, value, expiryInMinutes) {
const now = new Date();
const item = {
value: value,
expiry: now.getTime() + expiryInMinutes * 60 * 1000, // 转换为毫秒
};
localStorage.setItem(key, JSON.stringify(item));
}
2. 获取数据时检查是否过期
在获取数据时,检查当前时间是否超过了存储的时间戳。如果超过了,则清除数据并返回 null
。
function getLocalStorageWithExpiry(key) {
const itemStr = localStorage.getItem(key);
if (!itemStr) {
return null;
}
const item = JSON.parse(itemStr);
const now = new Date();
if (now.getTime() > item.expiry) {
// 如果数据已过期,清除它
localStorage.removeItem(key);
return null;
}
return item.value;
}
3. 使用示例
// 存储数据,设置5分钟后过期
setLocalStorageWithExpiry('myKey', 'myValue', 5);
// 获取数据
const value = getLocalStorageWithExpiry('myKey');
if (value) {
console.log('数据有效:', value);
} else {
console.log('数据已过期或不存在');
}
4. 自动清理过期数据
如果你希望定期清理过期的数据,可以使用 setInterval
定时器来定期检查并清理过期的 localStorage
数据。
function clearExpiredLocalStorage() {
Object.keys(localStorage).forEach(key => {
getLocalStorageWithExpiry(key); // 获取数据时会自动清理过期数据
});
}
// 每10分钟清理一次过期数据
setInterval(clearExpiredLocalStorage, 10 * 60 * 1000);
总结
通过这种方式,你可以为 localStorage
中的数据设置失效时间,并在数据过期时自动清除。这种方法适用于需要在一定时间后自动失效的场景,如用户登录状态、缓存数据等。