JavaScript中Promise的链式调用实现方式
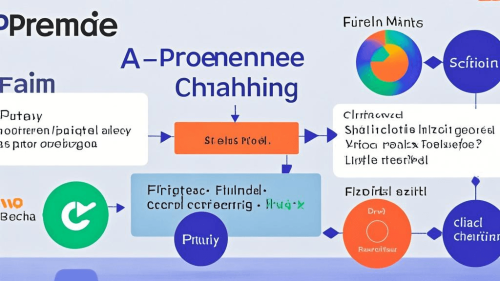
Promise 是 JavaScript 中用于处理异步操作的一种机制,它允许我们以链式调用的方式组织异步代码,使得代码更加清晰和易于维护。Promise 的链式调用是通过 then
方法实现的。以下是 Promise 实现链式调用的方式:
1. 基本链式调用
每个 then
方法都会返回一个新的 Promise 对象,因此可以在 then
方法后面继续调用 then
方法,形成链式调用。
const promise = new Promise((resolve, reject) => {
setTimeout(() => resolve(1), 1000);
});
promise
.then(result => {
console.log(result); // 1
return result * 2;
})
.then(result => {
console.log(result); // 2
return result * 2;
})
.then(result => {
console.log(result); // 4
});
2. 处理异步操作
在 then
方法中可以返回一个新的 Promise 对象,从而实现异步操作的链式调用。
const fetchData = () => {
return new Promise((resolve, reject) => {
setTimeout(() => resolve("Data fetched"), 1000);
});
};
fetchData()
.then(data => {
console.log(data); // "Data fetched"
return new Promise((resolve, reject) => {
setTimeout(() => resolve("Processed data"), 1000);
});
})
.then(processedData => {
console.log(processedData); // "Processed data"
});
3. 错误处理
在链式调用中,可以使用 catch
方法来捕获链中任何一个 then
方法抛出的错误。
const promise = new Promise((resolve, reject) => {
setTimeout(() => resolve(1), 1000);
});
promise
.then(result => {
console.log(result); // 1
throw new Error("Something went wrong");
})
.then(result => {
console.log(result); // 不会执行
})
.catch(error => {
console.error(error.message); // "Something went wrong"
});
4. finally
方法
finally
方法会在 Promise 链执行完毕后无论成功还是失败都会执行,通常用于清理操作。
const promise = new Promise((resolve, reject) => {
setTimeout(() => resolve(1), 1000);
});
promise
.then(result => {
console.log(result); // 1
return result * 2;
})
.then(result => {
console.log(result); // 2
})
.catch(error => {
console.error(error);
})
.finally(() => {
console.log("Promise chain finished");
});
5. 返回 Promise 的静态方法
Promise.resolve
和 Promise.reject
可以用于快速创建一个已解决或已拒绝的 Promise,从而在链式调用中使用。
Promise.resolve(1)
.then(result => {
console.log(result); // 1
return Promise.resolve(result * 2);
})
.then(result => {
console.log(result); // 2
return Promise.reject("Error occurred");
})
.catch(error => {
console.error(error); // "Error occurred"
});
6. 并行执行多个 Promise
Promise.all
和 Promise.race
可以用于并行执行多个 Promise,并在链式调用中处理结果。
const promise1 = Promise.resolve(1);
const promise2 = Promise.resolve(2);
const promise3 = Promise.resolve(3);
Promise.all([promise1, promise2, promise3])
.then(results => {
console.log(results); // [1, 2, 3]
})
.catch(error => {
console.error(error);
});
总结
Promise 的链式调用通过 then
方法实现,每个 then
方法返回一个新的 Promise 对象,从而可以继续调用 then
方法。通过 catch
方法可以捕获链中的错误,finally
方法用于执行最终的操作。Promise.resolve
和 Promise.reject
可以快速创建已解决或已拒绝的 Promise,而 Promise.all
和 Promise.race
可以用于并行执行多个 Promise。
这种链式调用的方式使得异步代码的组织更加清晰和易于维护,是现代 JavaScript 开发中的重要特性。