前端开发:使用两个队列实现栈的详细指南
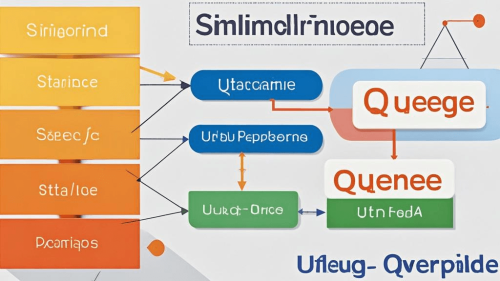
在前端开发中,虽然我们通常使用栈和队列来处理不同的场景,但有时我们可能需要用队列来实现栈的功能。栈是一种后进先出(LIFO)的数据结构,而队列是一种先进先出(FIFO)的数据结构。要实现栈的功能,我们需要通过队列的操作来模拟栈的行为。
使用两个队列实现栈
我们可以使用两个队列来模拟栈的行为。具体思路如下:
- 入栈操作:将元素插入到非空的队列中。
- 出栈操作:将非空队列中的元素依次出队并插入到另一个空队列中,直到只剩下一个元素,然后将这个元素出队并返回。
代码实现
以下是一个使用两个队列实现栈的示例代码:
class Queue {
constructor() {
this.items = [];
}
enqueue(element) {
this.items.push(element);
}
dequeue() {
if (this.isEmpty()) {
return "Queue is empty";
}
return this.items.shift();
}
isEmpty() {
return this.items.length === 0;
}
size() {
return this.items.length;
}
front() {
if (this.isEmpty()) {
return "Queue is empty";
}
return this.items[0];
}
}
class StackUsingQueues {
constructor() {
this.queue1 = new Queue();
this.queue2 = new Queue();
}
push(element) {
// 将元素插入到非空的队列中
if (this.queue1.isEmpty()) {
this.queue2.enqueue(element);
} else {
this.queue1.enqueue(element);
}
}
pop() {
if (this.isEmpty()) {
return "Stack is empty";
}
// 将非空队列中的元素依次出队并插入到另一个空队列中,直到只剩下一个元素
if (this.queue1.isEmpty()) {
while (this.queue2.size() > 1) {
this.queue1.enqueue(this.queue2.dequeue());
}
return this.queue2.dequeue();
} else {
while (this.queue1.size() > 1) {
this.queue2.enqueue(this.queue1.dequeue());
}
return this.queue1.dequeue();
}
}
isEmpty() {
return this.queue1.isEmpty() && this.queue2.isEmpty();
}
top() {
if (this.isEmpty()) {
return "Stack is empty";
}
// 返回非空队列的最后一个元素
if (this.queue1.isEmpty()) {
return this.queue2.front();
} else {
return this.queue1.front();
}
}
}
// 示例用法
const stack = new StackUsingQueues();
stack.push(1);
stack.push(2);
stack.push(3);
console.log(stack.pop()); // 输出 3
console.log(stack.pop()); // 输出 2
console.log(stack.top()); // 输出 1
解释
- Queue类:实现了一个简单的队列数据结构,支持入队、出队、判断是否为空、获取队列大小和获取队首元素的操作。
- StackUsingQueues类:使用两个队列来模拟栈的行为。
push
方法将元素插入到非空的队列中,pop
方法通过将非空队列中的元素转移到另一个队列中,直到只剩下一个元素,然后将其出队并返回。
复杂度分析
- 时间复杂度:
push
操作的时间复杂度为 O(1),因为只需要将元素插入到队列中。pop
操作的时间复杂度为 O(n),因为需要将 n-1 个元素从一个队列转移到另一个队列。
- 空间复杂度:O(n),因为需要使用两个队列来存储元素。
总结
通过使用两个队列,我们可以模拟栈的行为。虽然这种方法在时间复杂度上不如直接使用栈高效,但在某些特定场景下,这种实现方式可能会派上用场。在实际开发中,我们通常会直接使用栈数据结构,但在需要时,这种实现方式可以作为一个备选方案。