React 组件通信的常见方式及应用场景
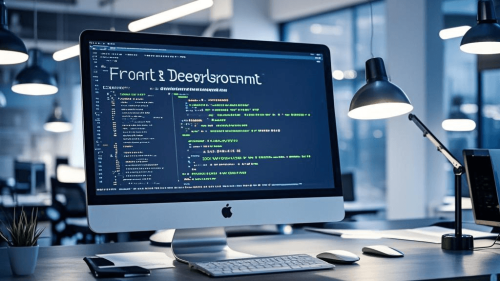
在 React 中,组件通信是一个核心概念,尤其是在构建复杂应用时。React 提供了多种方式来实现组件之间的通信,具体选择哪种方式取决于组件的层级关系和应用场景。以下是几种常见的 React 组件通信方式:
1. Props 传递
- 适用场景: 父组件向子组件传递数据。
- 实现方式: 父组件通过
props
将数据传递给子组件。 - 示例:
function ParentComponent() { const data = "Hello from Parent"; return <ChildComponent message={data} />; } function ChildComponent({ message }) { return <div>{message}</div>; }
2. 回调函数(Callback Functions)
- 适用场景: 子组件向父组件传递数据或触发父组件的某些行为。
- 实现方式: 父组件通过
props
传递一个回调函数给子组件,子组件调用该函数并将数据作为参数传递。 - 示例:
function ParentComponent() { const handleData = (data) => { console.log("Data from child:", data); }; return <ChildComponent onData={handleData} />; } function ChildComponent({ onData }) { const data = "Hello from Child"; return <button onClick={() => onData(data)}>Send Data</button>; }
3. Context API
- 适用场景: 跨层级组件通信,避免
props drilling
(即多层嵌套传递props
)。 - 实现方式: 使用
React.createContext
创建一个上下文,然后通过Provider
提供数据,子组件通过Consumer
或useContext
钩子来消费数据。 - 示例:
const MyContext = React.createContext(); function ParentComponent() { return ( <MyContext.Provider value="Hello from Context"> <ChildComponent /> </MyContext.Provider> ); } function ChildComponent() { const value = React.useContext(MyContext); return <div>{value}</div>; }
4. 状态管理库(如 Redux、MobX、Recoil)
- 适用场景: 全局状态管理,适用于大型应用或需要跨多个组件共享状态的场景。
- 实现方式: 使用状态管理库来集中管理应用的状态,组件通过订阅状态或派发动作来更新状态。
- 示例(以 Redux 为例):
// store.js import { createStore } from 'redux'; const initialState = { message: "Hello from Redux" }; function reducer(state = initialState, action) { switch (action.type) { case 'UPDATE_MESSAGE': return { ...state, message: action.payload }; default: return state; } } const store = createStore(reducer); // ParentComponent.js import { Provider } from 'react-redux'; import ChildComponent from './ChildComponent'; function ParentComponent() { return ( <Provider store={store}> <ChildComponent /> </Provider> ); } // ChildComponent.js import { useSelector, useDispatch } from 'react-redux'; function ChildComponent() { const message = useSelector((state) => state.message); const dispatch = useDispatch(); const updateMessage = () => { dispatch({ type: 'UPDATE_MESSAGE', payload: "Updated Message" }); }; return ( <div> <div>{message}</div> <button onClick={updateMessage}>Update Message</button> </div> ); }
5. 事件总线(Event Bus)
- 适用场景: 非父子组件之间的通信,或者组件层级较深且不适合使用
Context
的场景。 - 实现方式: 使用自定义事件系统或第三方库(如
EventEmitter
)来实现组件之间的通信。 - 示例:
import EventEmitter from 'events'; const eventBus = new EventEmitter(); function ComponentA() { const handleClick = () => { eventBus.emit('customEvent', 'Hello from ComponentA'); }; return <button onClick={handleClick}>Send Event</button>; } function ComponentB() { const [message, setMessage] = React.useState(''); React.useEffect(() => { const handleEvent = (data) => { setMessage(data); }; eventBus.on('customEvent', handleEvent); return () => { eventBus.off('customEvent', handleEvent); }; }, []); return <div>{message}</div>; }
6. Refs 和 Forwarding Refs
- 适用场景: 父组件需要直接访问子组件的 DOM 元素或实例。
- 实现方式: 使用
React.createRef
或useRef
创建ref
,并通过React.forwardRef
将ref
传递给子组件。 - 示例:
const ChildComponent = React.forwardRef((props, ref) => { return <input ref={ref} />; }); function ParentComponent() { const inputRef = React.useRef(); const focusInput = () => { inputRef.current.focus(); }; return ( <div> <ChildComponent ref={inputRef} /> <button onClick={focusInput}>Focus Input</button> </div> ); }
7. Portals
- 适用场景: 将子组件渲染到 DOM 树中的不同位置,通常用于模态框、弹出层等场景。
- 实现方式: 使用
ReactDOM.createPortal
将组件渲染到指定的 DOM 节点。 - 示例:
function Modal({ children }) { return ReactDOM.createPortal( <div className="modal">{children}</div>, document.getElementById('modal-root') ); } function ParentComponent() { return ( <div> <Modal>This is a modal</Modal> </div> ); }
8. 自定义 Hooks
- 适用场景: 封装可复用的逻辑,并在多个组件之间共享。
- 实现方式: 创建自定义 Hook,并在组件中使用它。
- 示例:
function useCounter(initialValue = 0) { const [count, setCount] = React.useState(initialValue); const increment = () => setCount(count + 1); return { count, increment }; } function ComponentA() { const { count, increment } = useCounter(); return ( <div> <div>{count}</div> <button onClick={increment}>Increment</button> </div> ); } function ComponentB() { const { count, increment } = useCounter(10); return ( <div> <div>{count}</div> <button onClick={increment}>Increment</button> </div> ); }
总结
React 提供了多种组件通信的方式,开发者应根据具体的应用场景选择最合适的方式。对于简单的父子组件通信,props
和回调函数是最常用的方式;对于跨层级或全局状态管理,Context API
或状态管理库(如 Redux)是更好的选择;而对于非父子组件之间的通信,事件总线或自定义 Hooks 可能更为合适。