React高阶组件技术解析
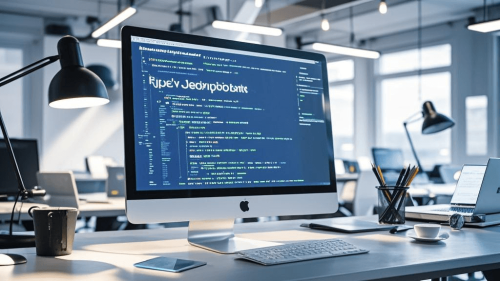
高阶组件(Higher-Order Component,HOC)是 React 中用于复用组件逻辑的一种高级技术。它本质上是一个函数,接受一个组件并返回一个新的组件。高阶组件并不是 React API 的一部分,而是基于 React 的组合特性衍生出来的一种设计模式。
高阶组件的定义
高阶组件是一个函数,其签名通常如下:
const EnhancedComponent = higherOrderComponent(WrappedComponent);
其中:
higherOrderComponent
是一个函数,接受一个组件作为参数。WrappedComponent
是被包装的组件。EnhancedComponent
是返回的新组件。
高阶组件的核心思想
高阶组件的核心思想是通过将组件作为参数传入一个函数,并在函数内部对组件进行增强或修改,从而实现逻辑的复用。它类似于高阶函数(Higher-Order Function),即函数可以作为参数传递或返回。
高阶组件的应用场景
-
逻辑复用:
- 当多个组件需要共享相同的逻辑(如数据获取、权限校验、日志记录等)时,可以将这些逻辑提取到高阶组件中,避免代码重复。
- 例如,一个用于获取用户信息的高阶组件:
function withUserData(WrappedComponent) { return class extends React.Component { state = { userData: null }; componentDidMount() { fetch('/api/user') .then(response => response.json()) .then(userData => this.setState({ userData })); } render() { return <WrappedComponent userData={this.state.userData} {...this.props} />; } }; }
-
条件渲染:
- 高阶组件可以根据某些条件决定是否渲染被包装的组件。
- 例如,一个用于权限校验的高阶组件:
function withAuth(WrappedComponent) { return class extends React.Component { render() { const isAuthenticated = checkAuth(); // 假设这是一个检查用户是否登录的函数 if (!isAuthenticated) { return <Redirect to="/login" />; } return <WrappedComponent {...this.props} />; } }; }
-
Props 增强:
- 高阶组件可以向被包装的组件注入额外的 props。
- 例如,一个用于注入主题的高阶组件:
function withTheme(WrappedComponent) { return class extends React.Component { render() { const theme = getCurrentTheme(); // 假设这是一个获取当前主题的函数 return <WrappedComponent theme={theme} {...this.props} />; } }; }
-
性能优化:
- 高阶组件可以用于实现性能优化,例如通过
React.memo
或shouldComponentUpdate
来避免不必要的渲染。 - 例如,一个用于优化渲染的高阶组件:
function withOptimization(WrappedComponent) { return React.memo(WrappedComponent); }
- 高阶组件可以用于实现性能优化,例如通过
-
调试和日志记录:
- 高阶组件可以用于在组件的生命周期中添加调试信息或日志记录。
- 例如,一个用于记录组件生命周期的高阶组件:
function withLogger(WrappedComponent) { return class extends React.Component { componentDidMount() { console.log(`${WrappedComponent.name} mounted`); } componentWillUnmount() { console.log(`${WrappedComponent.name} will unmount`); } render() { return <WrappedComponent {...this.props} />; } }; }
高阶组件的注意事项
-
避免修改原组件:
- 高阶组件应该通过组合的方式增强组件,而不是直接修改原组件的实现。
-
传递 Props:
- 高阶组件需要确保将所有的 props 传递给被包装的组件,避免丢失 props。
-
命名冲突:
- 高阶组件注入的 props 可能会与被包装组件的 props 发生命名冲突,需要注意命名空间的管理。
-
静态方法丢失:
- 高阶组件可能会导致被包装组件的静态方法丢失,可以通过
hoist-non-react-statics
库来解决。
- 高阶组件可能会导致被包装组件的静态方法丢失,可以通过
-
Refs 传递:
- 高阶组件默认不会传递 refs,如果需要传递 refs,可以使用
React.forwardRef
。
- 高阶组件默认不会传递 refs,如果需要传递 refs,可以使用
高阶组件与 Render Props 的对比
高阶组件和 Render Props 都是 React 中用于逻辑复用的模式,但它们有不同的适用场景:
- 高阶组件:更适合用于增强组件的行为或注入 props。
- Render Props:更适合用于动态渲染内容或共享状态。
总结
高阶组件是 React 中一种强大的模式,用于复用组件逻辑、增强组件功能以及实现条件渲染等。通过合理使用高阶组件,可以显著提高代码的复用性和可维护性。然而,在使用高阶组件时,也需要注意其潜在的问题,如命名冲突、静态方法丢失等。