JavaScript判断数组包含值的方法
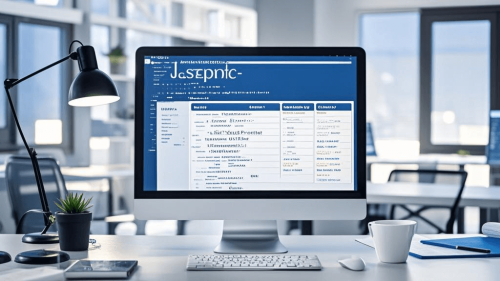
在 JavaScript 中,判断数组中是否包含某个值有多种方法,以下是几种常见的方式:
1. 使用 Array.prototype.includes()
方法
includes()
方法是最简洁的方式,它返回一个布尔值,表示数组是否包含指定的值。
const array = [1, 2, 3, 4, 5];
const value = 3;
if (array.includes(value)) {
console.log('数组包含该值');
} else {
console.log('数组不包含该值');
}
- 优点:语法简洁,易读。
- 缺点:不支持 IE 浏览器。
2. 使用 Array.prototype.indexOf()
方法
indexOf()
方法返回指定值在数组中的第一个索引,如果不存在则返回 -1
。
const array = [1, 2, 3, 4, 5];
const value = 3;
if (array.indexOf(value) !== -1) {
console.log('数组包含该值');
} else {
console.log('数组不包含该值');
}
- 优点:兼容性好,支持所有主流浏览器。
- 缺点:语法稍显冗长。
3. 使用 Array.prototype.find()
方法
find()
方法返回数组中满足提供的测试函数的第一个元素的值,否则返回 undefined
。
const array = [1, 2, 3, 4, 5];
const value = 3;
if (array.find(item => item === value)) {
console.log('数组包含该值');
} else {
console.log('数组不包含该值');
}
- 优点:可以处理复杂的查找逻辑。
- 缺点:对于简单的值查找,不如
includes()
或indexOf()
简洁。
4. 使用 Array.prototype.some()
方法
some()
方法测试数组中是否至少有一个元素通过了提供的测试函数。
const array = [1, 2, 3, 4, 5];
const value = 3;
if (array.some(item => item === value)) {
console.log('数组包含该值');
} else {
console.log('数组不包含该值');
}
- 优点:适用于复杂的条件判断。
- 缺点:对于简单的值查找,不如
includes()
简洁。
5. 使用 Set
数据结构
如果你需要频繁检查数组中是否包含某个值,可以考虑将数组转换为 Set
,因为 Set
的 has()
方法在查找时具有更高的性能。
const array = [1, 2, 3, 4, 5];
const set = new Set(array);
const value = 3;
if (set.has(value)) {
console.log('数组包含该值');
} else {
console.log('数组不包含该值');
}
- 优点:查找性能高,适合频繁查找的场景。
- 缺点:需要额外的内存空间来存储
Set
。
总结
- 对于简单的值查找,推荐使用
includes()
方法,因为它简洁且易读。 - 如果需要兼容旧版浏览器,可以使用
indexOf()
方法。 - 对于复杂的查找逻辑,可以使用
find()
或some()
方法。 - 如果需要频繁查找,考虑使用
Set
数据结构。
根据具体场景选择合适的方法,可以提高代码的可读性和性能。