React 项目集成应用 TypeScript 的步骤与实践
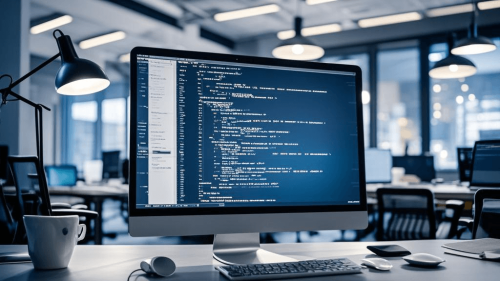
在 React 项目中应用 TypeScript 可以显著提升代码的可维护性和开发体验。以下是如何在 React 项目中集成和应用 TypeScript 的详细步骤和最佳实践:
1. 初始化项目
如果你是从零开始一个 React 项目,可以使用 create-react-app
并指定 TypeScript 模板:
npx create-react-app my-app --template typescript
这将自动配置好 TypeScript 环境,并生成必要的配置文件(如 tsconfig.json
)。
2. 配置 tsconfig.json
tsconfig.json
是 TypeScript 的配置文件,用于指定编译选项。以下是一些常见的配置项:
{
"compilerOptions": {
"target": "es5",
"lib": ["dom", "dom.iterable", "esnext"],
"allowJs": true,
"skipLibCheck": true,
"esModuleInterop": true,
"allowSyntheticDefaultImports": true,
"strict": true,
"forceConsistentCasingInFileNames": true,
"noFallthroughCasesInSwitch": true,
"module": "esnext",
"moduleResolution": "node",
"resolveJsonModule": true,
"isolatedModules": true,
"noEmit": true,
"jsx": "react-jsx"
},
"include": ["src"]
}
strict
: 启用所有严格的类型检查选项,建议开启以确保代码质量。jsx
: 指定 JSX 的处理方式,react-jsx
是 React 17+ 的推荐配置。
3. 定义组件 Props 和 State
使用 TypeScript 定义组件的 props
和 state
类型,可以避免常见的类型错误。
函数组件
import React from 'react';
interface MyComponentProps {
name: string;
age: number;
}
const MyComponent: React.FC<MyComponentProps> = ({ name, age }) => {
return (
<div>
<p>Name: {name}</p>
<p>Age: {age}</p>
</div>
);
};
export default MyComponent;
类组件
import React, { Component } from 'react';
interface MyComponentProps {
name: string;
age: number;
}
interface MyComponentState {
count: number;
}
class MyComponent extends Component<MyComponentProps, MyComponentState> {
state: MyComponentState = {
count: 0,
};
render() {
const { name, age } = this.props;
const { count } = this.state;
return (
<div>
<p>Name: {name}</p>
<p>Age: {age}</p>
<p>Count: {count}</p>
</div>
);
}
}
export default MyComponent;
4. 使用 Hooks 和 TypeScript
TypeScript 可以很好地与 React Hooks 结合使用。
useState
import React, { useState } from 'react';
const Counter: React.FC = () => {
const [count, setCount] = useState<number>(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default Counter;
useEffect
import React, { useEffect, useState } from 'react';
const DataFetcher: React.FC = () => {
const [data, setData] = useState<any>(null);
useEffect(() => {
fetch('/api/data')
.then(response => response.json())
.then(data => setData(data));
}, []);
return (
<div>
{data ? <pre>{JSON.stringify(data, null, 2)}</pre> : 'Loading...'}
</div>
);
};
export default DataFetcher;
5. 处理事件
TypeScript 可以帮助你更好地处理事件类型。
import React from 'react';
const EventHandler: React.FC = () => {
const handleClick = (event: React.MouseEvent<HTMLButtonElement>) => {
console.log('Button clicked', event.currentTarget);
};
return (
<button onClick={handleClick}>Click me</button>
);
};
export default EventHandler;
6. 使用第三方库
许多第三方库都提供了 TypeScript 类型定义。如果库没有自带类型定义,你可以通过 @types
安装。
npm install --save @types/react-router-dom
7. 类型推断与类型断言
TypeScript 的类型推断非常强大,但在某些情况下你可能需要手动指定类型。
const user = {
name: 'John',
age: 30,
};
// 类型推断
const name = user.name; // string
// 类型断言
const age = user.age as number;
8. 泛型组件
TypeScript 的泛型可以用于创建更灵活的组件。
interface ListProps<T> {
items: T[];
renderItem: (item: T) => React.ReactNode;
}
function List<T>({ items, renderItem }: ListProps<T>) {
return (
<ul>
{items.map((item, index) => (
<li key={index}>{renderItem(item)}</li>
))}
</ul>
);
}
export default List;
9. 高级类型工具
TypeScript 提供了许多高级类型工具,如 Partial
, Pick
, Omit
等,可以帮助你更灵活地定义类型。
interface User {
id: number;
name: string;
age: number;
email: string;
}
type PartialUser = Partial<User>; // 所有属性变为可选
type UserName = Pick<User, 'name'>; // 只选择 'name' 属性
type UserWithoutEmail = Omit<User, 'email'>; // 排除 'email' 属性
10. 测试与调试
TypeScript 的类型检查可以在编译时捕获许多错误,但测试仍然是必要的。使用 Jest 和 React Testing Library 进行单元测试和集成测试。
import { render, screen } from '@testing-library/react';
import MyComponent from './MyComponent';
test('renders name and age', () => {
render(<MyComponent name="John" age={30} />);
expect(screen.getByText(/Name: John/i)).toBeInTheDocument();
expect(screen.getByText(/Age: 30/i)).toBeInTheDocument();
});
总结
在 React 项目中应用 TypeScript 可以显著提升代码质量和开发效率。通过合理配置 tsconfig.json
、定义组件类型、使用 Hooks 和高级类型工具,你可以构建出类型安全且易于维护的 React 应用。