TypeScript项目如何使用node_modules全局类型包
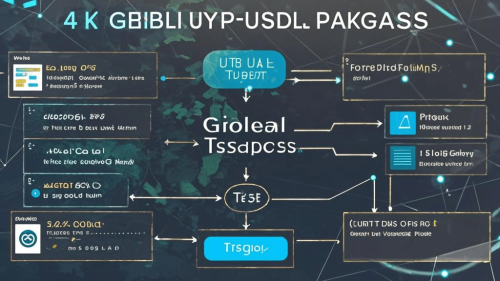
在 TypeScript 项目中,如果你想使用 node_modules
中定义的全局类型包(例如 @types
包或其他第三方库的类型定义),通常不需要手动将这些类型定义复制到 src
目录下。TypeScript 编译器会自动解析 node_modules
中的类型定义。
以下是几种常见的方式来使用 node_modules
中的全局类型包:
1. 自动解析 @types
包
TypeScript 会自动查找 node_modules/@types
目录下的类型定义。如果你安装了一个带有类型定义的包(例如 @types/lodash
),TypeScript 会自动识别并使用这些类型。
例如,安装 lodash
和它的类型定义:
npm install lodash
npm install --save-dev @types/lodash
然后在你的代码中直接使用:
import _ from 'lodash';
const result = _.map([1, 2, 3], n => n * 2);
console.log(result);
2. 使用 typeRoots
配置
如果你有自定义的类型定义目录,或者你想指定 TypeScript 查找类型定义的路径,可以在 tsconfig.json
中配置 typeRoots
。
{
"compilerOptions": {
"typeRoots": ["./node_modules/@types", "./src/types"]
}
}
这样,TypeScript 会优先查找 node_modules/@types
和 src/types
目录下的类型定义。
3. 使用 types
配置
如果你只想包含特定的类型定义包,可以在 tsconfig.json
中使用 types
配置。
{
"compilerOptions": {
"types": ["lodash", "jquery"]
}
}
这样,TypeScript 只会包含 lodash
和 jquery
的类型定义,忽略其他 @types
包。
4. 手动引入类型定义
如果你有一个全局类型定义文件(例如 .d.ts
文件),你可以将其放在 src
目录下,并在 tsconfig.json
中通过 include
或 files
配置来包含它。
例如,假设你有一个 src/types/custom.d.ts
文件:
declare module 'custom-module' {
export function doSomething(): void;
}
然后在 tsconfig.json
中确保包含这个文件:
{
"include": ["src/**/*"]
}
这样,TypeScript 会自动识别 src/types/custom.d.ts
中的类型定义。
5. 使用 paths
配置别名
如果你想要为某些模块设置别名,可以在 tsconfig.json
中使用 paths
配置。
{
"compilerOptions": {
"baseUrl": ".",
"paths": {
"@utils/*": ["src/utils/*"]
}
}
}
然后在代码中可以使用别名导入模块:
import { someUtil } from '@utils/someUtil';
总结
通常情况下,TypeScript 会自动解析 node_modules
中的类型定义,你不需要手动复制这些类型定义到 src
目录下。如果你有特殊需求,可以通过 typeRoots
、types
、paths
等配置来定制 TypeScript 的类型解析行为。