JavaScript中bind方法的模拟实现与原理解析
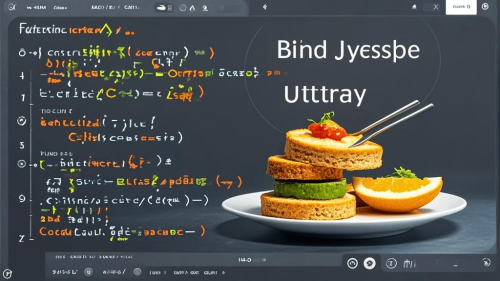
bind
是 JavaScript 中的一个内置方法,用于创建一个新的函数,并将指定的 this
值绑定到该函数。bind
还允许你预先设置函数的参数(即部分应用参数)。我们可以通过模拟实现 bind
来更好地理解它的工作原理。
下面是一个模拟 bind
的实现:
Function.prototype.myBind = function(context, ...args) {
// 保存当前的 this,即调用 myBind 的函数
const fn = this;
// 返回一个新的函数
return function(...innerArgs) {
// 在调用时,将 this 绑定到 context,并合并参数
return fn.apply(context, args.concat(innerArgs));
};
};
解释:
-
Function.prototype.myBind
: 我们将myBind
方法添加到Function
的原型上,这样所有的函数都可以调用myBind
。 -
context
: 这是你想要绑定的this
值。 -
...args
: 这是传递给myBind
的预先设置的参数。 -
fn = this
: 在myBind
方法内部,this
指向调用myBind
的函数。我们将这个函数保存到fn
变量中。 -
返回一个新函数:
myBind
返回一个新的函数,这个函数在被调用时会将this
绑定到context
,并且会将预先设置的参数和调用时传入的参数合并。 -
fn.apply(context, args.concat(innerArgs))
: 使用apply
方法调用原始函数fn
,并将this
绑定到context
。args.concat(innerArgs)
将预先设置的参数和调用时传入的参数合并。
使用示例:
const person = {
name: 'Alice',
greet: function(greeting, punctuation) {
console.log(`${greeting}, ${this.name}${punctuation}`);
}
};
const greet = person.greet.myBind(person, 'Hello');
greet('!'); // 输出: "Hello, Alice!"
在这个例子中,myBind
将 this
绑定到 person
对象,并预先设置了 'Hello'
作为第一个参数。当调用 greet('!')
时,'!'
作为第二个参数传入,最终输出 "Hello, Alice!"
。
总结:
通过模拟 bind
的实现,我们可以更好地理解 JavaScript 中的 this
绑定和函数柯里化(currying)的概念。这个实现虽然简单,但涵盖了 bind
的核心功能。