JavaScript中BigInt的使用方法与注意事项
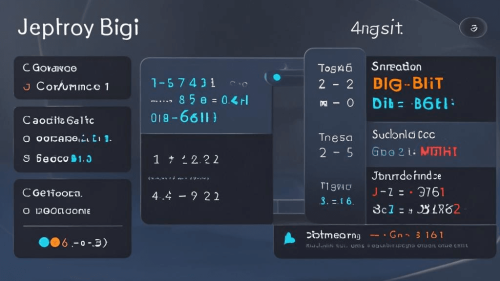
在 JavaScript 中,BigInt
是一种内置对象,用于表示大于 2^53 - 1
(即 Number.MAX_SAFE_INTEGER
)的整数。BigInt
可以表示任意大的整数,并且支持与普通整数类似的算术操作。
创建 BigInt
的方法
-
使用
BigInt
构造函数:
你可以通过将整数或字符串传递给BigInt
构造函数来创建一个BigInt
。const bigIntFromNumber = BigInt(12345678901234567890); const bigIntFromString = BigInt("12345678901234567890"); console.log(bigIntFromNumber); // 12345678901234567890n console.log(bigIntFromString); // 12345678901234567890n
-
使用
n
后缀:
你可以在整数后面直接加上n
后缀来创建一个BigInt
。const bigIntWithSuffix = 12345678901234567890n; console.log(bigIntWithSuffix); // 12345678901234567890n
使用 BigInt
BigInt
支持与普通整数类似的算术操作,如加法、减法、乘法和除法。需要注意的是,BigInt
不能与普通 Number
类型直接混合运算,必须先将 Number
转换为 BigInt
。
const a = 12345678901234567890n;
const b = 98765432109876543210n;
// 加法
const sum = a + b;
console.log(sum); // 111111111011111111100n
// 减法
const difference = b - a;
console.log(difference); // 86419753208641975320n
// 乘法
const product = a * b;
console.log(product); // 1219326311370217952237463801111263526900n
// 除法
const quotient = b / a;
console.log(quotient); // 8n
// 取余
const remainder = b % a;
console.log(remainder); // 8641975320864197530n
注意事项
-
类型转换:
BigInt
不能与Number
直接混合运算,必须显式转换。const bigInt = 123n; const number = 456; // 错误:不能直接混合运算 // const result = bigInt + number; // 正确:显式转换 const result = bigInt + BigInt(number); console.log(result); // 579n
-
比较操作:
BigInt
可以与Number
进行比较操作,但需要注意类型转换。const bigInt = 123n; const number = 123; console.log(bigInt == number); // true console.log(bigInt === number); // false
-
JSON 序列化:
BigInt
不能直接序列化为 JSON,需要手动处理。const data = { bigIntValue: 12345678901234567890n }; const jsonString = JSON.stringify(data, (key, value) => typeof value === 'bigint' ? value.toString() : value ); console.log(jsonString); // {"bigIntValue":"12345678901234567890"}
总结
BigInt
是 JavaScript 中处理大整数的重要工具,适用于需要处理超出 Number
类型范围的整数场景。通过 BigInt
构造函数或 n
后缀可以轻松创建 BigInt
,并且支持常见的算术操作。在使用时需要注意类型转换和 JSON 序列化等细节。