JavaScript中typeof操作符的使用场景与局限性
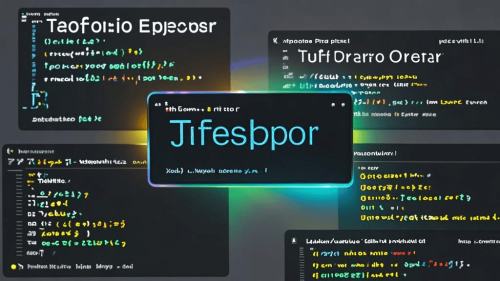
typeof
是 JavaScript 中的一个操作符,用于检测变量的数据类型。然而,它并不是在所有情况下都能正确判断类型。以下是一些 typeof
的使用场景及其局限性:
1. 基本类型
对于基本类型(如 number
, string
, boolean
, undefined
),typeof
通常能正确判断类型:
typeof 42; // "number"
typeof "hello"; // "string"
typeof true; // "boolean"
typeof undefined; // "undefined"
2. null
typeof null
返回 "object"
,这是一个历史遗留问题,实际上 null
是一个原始值,而不是对象:
typeof null; // "object"
3. 对象类型
对于对象类型(如 object
, array
, function
),typeof
的行为如下:
-
对于普通对象和数组,
typeof
返回"object"
:typeof {}; // "object" typeof []; // "object"
-
对于函数,
typeof
返回"function"
:typeof function() {}; // "function"
4. Symbol
对于 Symbol
类型,typeof
返回 "symbol"
:
typeof Symbol(); // "symbol"
5. BigInt
对于 BigInt
类型,typeof
返回 "bigint"
:
typeof 42n; // "bigint"
6. 其他情况
对于其他情况,如 NaN
,typeof
返回 "number"
:
typeof NaN; // "number"
总结
typeof
在大多数情况下可以正确判断基本类型,但在处理 null
和对象类型时存在局限性。为了更准确地判断类型,可以使用以下方法:
Object.prototype.toString.call()
:可以更准确地判断对象的类型。instanceof
:用于判断对象是否是某个构造函数的实例。Array.isArray()
:用于判断一个变量是否是数组。
例如:
Object.prototype.toString.call(null); // "[object Null]"
Object.prototype.toString.call([]); // "[object Array]"
[] instanceof Array; // true
Array.isArray([]); // true
在实际开发中,根据具体需求选择合适的类型判断方法是非常重要的。