JavaScript继承方式全解析
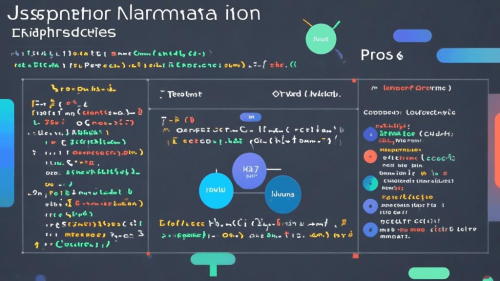
在 JavaScript 中,实现继承的方式有多种,每种方式都有其特点和适用场景。以下是几种常见的实现继承的方式:
1. 原型链继承
原型链继承是 JavaScript 中最基本的继承方式。通过将子类的原型对象指向父类的实例,子类就可以继承父类的属性和方法。
function Parent() {
this.name = 'Parent';
}
Parent.prototype.sayName = function() {
console.log(this.name);
};
function Child() {
this.name = 'Child';
}
// 继承
Child.prototype = new Parent();
const child = new Child();
child.sayName(); // 输出: Child
缺点:
- 所有子类实例共享同一个父类实例的引用属性,可能会导致意外的修改。
- 无法向父类构造函数传递参数。
2. 构造函数继承(借用构造函数)
通过在子类构造函数中调用父类构造函数,可以实现继承。
function Parent(name) {
this.name = name;
}
Parent.prototype.sayName = function() {
console.log(this.name);
};
function Child(name) {
Parent.call(this, name); // 调用父类构造函数
}
const child = new Child('Child');
console.log(child.name); // 输出: Child
child.sayName(); // 报错: child.sayName is not a function
缺点:
- 无法继承父类原型上的方法。
3. 组合继承(原型链 + 构造函数)
组合继承结合了原型链继承和构造函数继承的优点,既能够继承父类的实例属性,也能够继承父类原型上的方法。
function Parent(name) {
this.name = name;
}
Parent.prototype.sayName = function() {
console.log(this.name);
};
function Child(name) {
Parent.call(this, name); // 继承实例属性
}
// 继承原型方法
Child.prototype = new Parent();
Child.prototype.constructor = Child;
const child = new Child('Child');
child.sayName(); // 输出: Child
缺点:
- 父类构造函数被调用了两次,可能会导致性能问题。
4. 原型式继承
原型式继承通过创建一个临时构造函数,并将其原型指向父类对象,从而实现继承。
function createObject(obj) {
function F() {}
F.prototype = obj;
return new F();
}
const parent = {
name: 'Parent',
sayName: function() {
console.log(this.name);
}
};
const child = createObject(parent);
child.name = 'Child';
child.sayName(); // 输出: Child
缺点:
- 与原型链继承类似,所有子类实例共享同一个父类对象的引用属性。
5. 寄生式继承
寄生式继承在原型式继承的基础上,增强了对象的功能。
function createObject(obj) {
function F() {}
F.prototype = obj;
return new F();
}
function createChild(parent) {
const child = createObject(parent);
child.sayHello = function() {
console.log('Hello');
};
return child;
}
const parent = {
name: 'Parent',
sayName: function() {
console.log(this.name);
}
};
const child = createChild(parent);
child.name = 'Child';
child.sayName(); // 输出: Child
child.sayHello(); // 输出: Hello
缺点:
- 与原型式继承类似,所有子类实例共享同一个父类对象的引用属性。
6. 寄生组合式继承
寄生组合式继承是组合继承的优化版本,解决了组合继承中父类构造函数被调用两次的问题。
function inheritPrototype(child, parent) {
const prototype = Object.create(parent.prototype); // 创建父类原型的副本
prototype.constructor = child; // 修正constructor指向
child.prototype = prototype; // 将副本赋值给子类原型
}
function Parent(name) {
this.name = name;
}
Parent.prototype.sayName = function() {
console.log(this.name);
};
function Child(name) {
Parent.call(this, name); // 继承实例属性
}
inheritPrototype(Child, Parent);
const child = new Child('Child');
child.sayName(); // 输出: Child
优点:
- 只调用一次父类构造函数,避免了组合继承的性能问题。
- 保留了原型链的完整性。
7. ES6 Class 继承
ES6 引入了 class
和 extends
关键字,使得继承更加简洁和直观。
class Parent {
constructor(name) {
this.name = name;
}
sayName() {
console.log(this.name);
}
}
class Child extends Parent {
constructor(name) {
super(name); // 调用父类构造函数
}
}
const child = new Child('Child');
child.sayName(); // 输出: Child
优点:
- 语法简洁,易于理解。
- 支持
super
关键字调用父类方法。
总结
- 原型链继承:简单但存在共享引用问题。
- 构造函数继承:无法继承原型方法。
- 组合继承:结合了原型链和构造函数的优点,但父类构造函数被调用两次。
- 原型式继承:适用于简单对象继承。
- 寄生式继承:增强了原型式继承的功能。
- 寄生组合式继承:最优化的继承方式,解决了组合继承的性能问题。
- ES6 Class 继承:现代 JavaScript 推荐的方式,语法简洁且功能强大。
在实际开发中,推荐使用 ES6 Class 继承 或 寄生组合式继承,它们是最为高效和安全的继承方式。