项目中减少if-else语句使用的策略
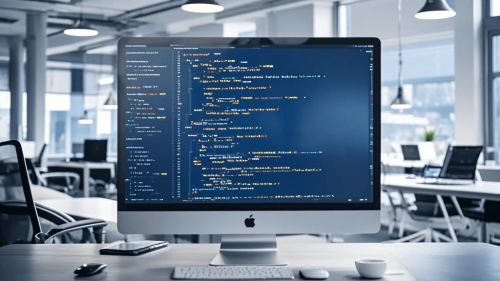
在项目中减少 if-else
语句的使用,不仅可以提高代码的可读性和可维护性,还能降低代码的复杂度。以下是一些常见的策略和最佳实践:
1. 使用多态(Polymorphism)
通过面向对象编程中的多态特性,可以将条件逻辑分散到不同的类中,从而减少 if-else
的使用。
class Animal {
speak() {
throw new Error('This method must be overridden');
}
}
class Dog extends Animal {
speak() {
return 'Woof!';
}
}
class Cat extends Animal {
speak() {
return 'Meow!';
}
}
const animals = [new Dog(), new Cat()];
animals.forEach(animal => console.log(animal.speak()));
2. 使用策略模式(Strategy Pattern)
策略模式允许你定义一系列算法,并将它们封装在独立的类中,使得它们可以互换使用。
const strategies = {
add: (a, b) => a + b,
subtract: (a, b) => a - b,
multiply: (a, b) => a * b,
divide: (a, b) => a / b,
};
function calculate(strategy, a, b) {
return strategies[strategy](a, b);
}
console.log(calculate('add', 5, 3)); // 8
3. 使用查找表(Lookup Table)
通过将条件逻辑映射到一个对象或数组中,可以减少 if-else
的使用。
const actions = {
'case1': () => console.log('Action for case 1'),
'case2': () => console.log('Action for case 2'),
'default': () => console.log('Default action'),
};
function performAction(case) {
const action = actions[case] || actions['default'];
action();
}
performAction('case1'); // Action for case 1
4. 使用函数式编程中的模式匹配
在函数式编程中,模式匹配是一种强大的工具,可以替代复杂的 if-else
结构。
const match = (value, patterns) => {
const pattern = patterns[value] || patterns.default;
return pattern();
};
const result = match('case1', {
'case1': () => 'Result for case 1',
'case2': () => 'Result for case 2',
'default': () => 'Default result',
});
console.log(result); // Result for case 1
5. 使用三元运算符
对于简单的条件判断,可以使用三元运算符来替代 if-else
。
const result = condition ? 'True' : 'False';
6. 使用短路逻辑
在某些情况下,可以使用逻辑运算符 &&
和 ||
来简化条件判断。
const result = condition && 'True' || 'False';
7. 使用早期返回(Early Return)
通过提前返回,可以减少嵌套的 if-else
结构。
function processData(data) {
if (!data) return;
// Process data
}
8. 使用状态机(State Machine)
对于复杂的状态转换逻辑,可以使用状态机来替代 if-else
。
const stateMachine = {
state: 'idle',
transitions: {
idle: {
start: () => {
console.log('Starting...');
this.state = 'running';
},
},
running: {
stop: () => {
console.log('Stopping...');
this.state = 'idle';
},
},
},
dispatch(action) {
const transition = this.transitions[this.state][action];
if (transition) transition();
},
};
stateMachine.dispatch('start'); // Starting...
stateMachine.dispatch('stop'); // Stopping...
9. 使用函数组合
通过将条件逻辑分解为多个小函数,可以减少 if-else
的使用。
const isEven = num => num % 2 === 0;
const isPositive = num => num > 0;
const checkNumber = num => {
if (isEven(num) && isPositive(num)) {
return 'Even and Positive';
}
return 'Other';
};
console.log(checkNumber(4)); // Even and Positive
10. 使用设计模式
某些设计模式(如责任链模式、命令模式等)可以帮助减少 if-else
的使用。
class Handler {
constructor() {
this.nextHandler = null;
}
setNext(handler) {
this.nextHandler = handler;
}
handle(request) {
if (this.nextHandler) {
return this.nextHandler.handle(request);
}
return null;
}
}
class ConcreteHandlerA extends Handler {
handle(request) {
if (request === 'A') {
return 'Handled by A';
}
return super.handle(request);
}
}
class ConcreteHandlerB extends Handler {
handle(request) {
if (request === 'B') {
return 'Handled by B';
}
return super.handle(request);
}
}
const handlerA = new ConcreteHandlerA();
const handlerB = new ConcreteHandlerB();
handlerA.setNext(handlerB);
console.log(handlerA.handle('B')); // Handled by B
通过以上方法,你可以有效地减少项目中的 if-else
语句,使代码更加简洁、可读和易于维护。