JavaScript 中 this 关键字在不同上下文中的行为
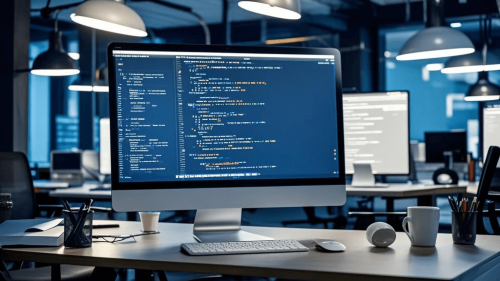
在 JavaScript 中,this
是一个特殊的关键字,它在不同的上下文中指向不同的对象。理解 this
的行为对于编写高质量的 JavaScript 代码至关重要。以下是 this
在不同上下文中的行为:
1. 全局上下文
在全局执行上下文中(即在任何函数外部),this
指向全局对象。在浏览器中,全局对象是 window
,而在 Node.js 中,全局对象是 global
。
console.log(this); // 在浏览器中输出: Window {...}
2. 函数上下文
在函数内部,this
的值取决于函数是如何被调用的。
- 普通函数调用:在非严格模式下,
this
指向全局对象(在浏览器中是window
)。在严格模式下,this
是undefined
。
function foo() {
console.log(this);
}
foo(); // 在非严格模式下输出: Window {...}
- 方法调用:当函数作为对象的方法被调用时,
this
指向调用该方法的对象。
const obj = {
name: 'Alice',
greet: function() {
console.log(this.name);
}
};
obj.greet(); // 输出: Alice
3. 构造函数上下文
当函数作为构造函数使用(即使用 new
关键字调用)时,this
指向新创建的对象。
function Person(name) {
this.name = name;
}
const person = new Person('Bob');
console.log(person.name); // 输出: Bob
4. 箭头函数
箭头函数没有自己的 this
,它会捕获其所在上下文的 this
值。这意味着箭头函数中的 this
是在定义时确定的,而不是在调用时确定的。
const obj = {
name: 'Alice',
greet: function() {
setTimeout(() => {
console.log(this.name);
}, 100);
}
};
obj.greet(); // 输出: Alice
5. 显式绑定
你可以使用 call
、apply
或 bind
来显式地设置 this
的值。
call
和apply
:立即调用函数,并显式指定this
的值。
function greet() {
console.log(this.name);
}
const obj = { name: 'Alice' };
greet.call(obj); // 输出: Alice
bind
:返回一个新函数,并将this
绑定到指定的对象。
function greet() {
console.log(this.name);
}
const obj = { name: 'Alice' };
const boundGreet = greet.bind(obj);
boundGreet(); // 输出: Alice
6. 事件处理函数
在 DOM 事件处理函数中,this
通常指向触发事件的元素。
document.querySelector('button').addEventListener('click', function() {
console.log(this); // 输出: <button>...</button>
});
7. 类上下文
在类的方法中,this
指向类的实例。
class Person {
constructor(name) {
this.name = name;
}
greet() {
console.log(this.name);
}
}
const person = new Person('Charlie');
person.greet(); // 输出: Charlie
总结
this
的行为在 JavaScript 中是非常灵活的,它的值取决于函数的调用方式。理解 this
的绑定规则对于避免常见的错误和编写可维护的代码至关重要。在实际开发中,合理使用箭头函数、bind
、call
和 apply
可以帮助你更好地控制 this
的指向。