前端组件渲染效率提升与避免不必要render的方法
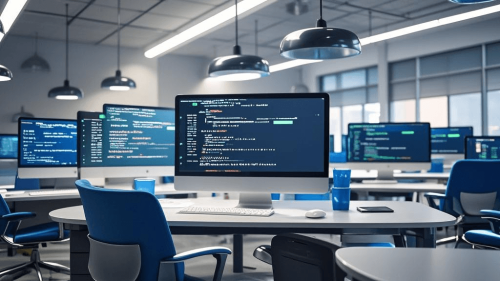
在前端开发中,提高组件渲染效率并避免不必要的 render
是优化应用性能的关键。以下是一些常见的方法和最佳实践:
1. 使用 React.memo
或 PureComponent
React.memo
:用于函数组件,它会浅比较组件的props
,只有在props
发生变化时才会重新渲染组件。const MyComponent = React.memo(({ prop1, prop2 }) => { return <div>{prop1} {prop2}</div>; });
PureComponent
:用于类组件,它会自动对props
和state
进行浅比较,避免不必要的渲染。class MyComponent extends React.PureComponent { render() { return <div>{this.props.prop1} {this.props.prop2}</div>; } }
2. 避免在 render
方法中创建新的对象或函数
- 在
render
方法中创建新的对象或函数会导致每次渲染时都生成新的引用,从而触发子组件的重新渲染。 - 解决方案是将这些对象或函数移到组件外部,或者使用
useCallback
和useMemo
来缓存它们。
3. 使用 useCallback
和 useMemo
useCallback
:用于缓存函数,避免在每次渲染时创建新的函数引用。const handleClick = React.useCallback(() => { console.log('Clicked'); }, []);
useMemo
:用于缓存计算结果,避免在每次渲染时重新计算。const memoizedValue = React.useMemo(() => computeExpensiveValue(a, b), [a, b]);
4. 避免不必要的 state
更新
- 只有在
state
或props
发生变化时才更新组件。避免在componentDidUpdate
或useEffect
中无意义地更新state
。
5. 使用 key
属性优化列表渲染
- 在渲染列表时,为每个列表项提供一个唯一的
key
属性,帮助 React 识别哪些项发生了变化、添加或删除,从而减少不必要的 DOM 操作。const items = data.map(item => <li key={item.id}>{item.name}</li>);
6. 使用 shouldComponentUpdate
或 React.memo
自定义比较逻辑
- 在类组件中,可以通过
shouldComponentUpdate
方法自定义比较逻辑,决定是否重新渲染组件。class MyComponent extends React.Component { shouldComponentUpdate(nextProps, nextState) { return nextProps.prop1 !== this.props.prop1; } render() { return <div>{this.props.prop1}</div>; } }
- 在函数组件中,可以通过
React.memo
的第二个参数自定义比较逻辑。const MyComponent = React.memo(({ prop1, prop2 }) => { return <div>{prop1} {prop2}</div>; }, (prevProps, nextProps) => { return prevProps.prop1 === nextProps.prop1; });
7. 使用 React.lazy
和 Suspense
进行代码分割
- 通过
React.lazy
和Suspense
实现按需加载组件,减少初始加载时的渲染负担。const LazyComponent = React.lazy(() => import('./LazyComponent')); function MyComponent() { return ( <React.Suspense fallback={<div>Loading...</div>}> <LazyComponent /> </React.Suspense> ); }
8. 使用 Context
时避免不必要的渲染
- 当使用
Context
时,避免将整个组件树包裹在Provider
中,因为Context
的变化会导致所有消费该Context
的组件重新渲染。可以通过将Context
拆分为多个小的Context
来减少不必要的渲染。
9. 使用 React.Fragment
减少不必要的 DOM 节点
- 使用
React.Fragment
可以减少不必要的 DOM 节点,从而减少渲染开销。function MyComponent() { return ( <React.Fragment> <div>Item 1</div> <div>Item 2</div> </React.Fragment> ); }
10. 使用 useReducer
替代 useState
管理复杂状态
- 当状态逻辑复杂时,使用
useReducer
可以更好地管理状态更新,避免不必要的渲染。const [state, dispatch] = React.useReducer(reducer, initialState);
11. 优化 CSS 和样式计算
- 避免在组件中使用复杂的 CSS 选择器或频繁的样式计算,这会影响渲染性能。可以使用 CSS-in-JS 库(如
styled-components
)来优化样式管理。
12. 使用 React.PureComponent
或 React.memo
优化高阶组件(HOC)
- 如果使用高阶组件,确保高阶组件本身是
PureComponent
或使用React.memo
进行优化,以避免不必要的渲染。
13. 使用 React DevTools
进行性能分析
- 使用
React DevTools
的Profiler
工具来分析组件的渲染性能,找出性能瓶颈并进行优化。
14. 避免在 render
方法中进行复杂的计算
- 将复杂的计算逻辑移到
useEffect
或useMemo
中,避免在每次渲染时都执行这些计算。
15. 使用 React.StrictMode
检测潜在问题
- 在开发环境中使用
React.StrictMode
可以帮助检测潜在的性能问题和副作用。
通过以上方法,可以显著提高组件的渲染效率,并避免不必要的 render
,从而提升应用的性能。