React事件绑定全解析
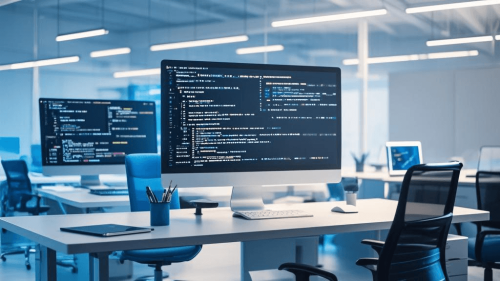
在 React 中,事件绑定是处理用户交互的重要部分。React 提供了一种类似于原生 DOM 事件处理的方式,但有一些关键的区别和最佳实践。以下是关于 React 事件绑定的详细说明:
1. 基本语法
在 React 中,事件处理函数通常通过 JSX 直接绑定到元素上。事件名称采用驼峰命名法(camelCase),例如 onClick
、onChange
等。
function MyComponent() {
const handleClick = () => {
console.log('Button clicked!');
};
return (
<button onClick={handleClick}>
Click Me
</button>
);
}
2. 事件对象
React 的事件处理函数会自动接收一个合成事件对象(SyntheticEvent
),它是 React 对原生浏览器事件的封装。这个对象与原生事件对象类似,但具有跨浏览器一致性。
function MyComponent() {
const handleClick = (event) => {
console.log('Event:', event);
console.log('Button clicked!');
};
return (
<button onClick={handleClick}>
Click Me
</button>
);
}
3. 传递参数
如果你需要在事件处理函数中传递额外的参数,可以使用箭头函数或 bind
方法。
使用箭头函数:
function MyComponent() {
const handleClick = (message) => {
console.log(message);
};
return (
<button onClick={() => handleClick('Hello, World!')}>
Click Me
</button>
);
}
使用 bind
方法:
function MyComponent() {
const handleClick = function(message) {
console.log(message);
};
return (
<button onClick={handleClick.bind(this, 'Hello, World!')}>
Click Me
</button>
);
}
4. 类组件中的事件绑定
在类组件中,事件处理函数通常作为类的方法定义。为了确保 this
的正确绑定,通常需要在构造函数中使用 bind
方法,或者使用箭头函数。
使用 bind
方法:
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
console.log('Button clicked!');
}
render() {
return (
<button onClick={this.handleClick}>
Click Me
</button>
);
}
}
使用箭头函数:
class MyComponent extends React.Component {
handleClick = () => {
console.log('Button clicked!');
};
render() {
return (
<button onClick={this.handleClick}>
Click Me
</button>
);
}
}
5. 阻止默认行为和事件冒泡
你可以使用 event.preventDefault()
来阻止默认行为,使用 event.stopPropagation()
来阻止事件冒泡。
function MyComponent() {
const handleClick = (event) => {
event.preventDefault();
event.stopPropagation();
console.log('Button clicked!');
};
return (
<a href="https://example.com" onClick={handleClick}>
Click Me
</a>
);
}
6. 性能优化
在函数组件中,如果事件处理函数在每次渲染时都重新创建,可能会导致不必要的重新渲染。为了避免这种情况,可以使用 useCallback
钩子来缓存事件处理函数。
import React, { useCallback } from 'react';
function MyComponent() {
const handleClick = useCallback(() => {
console.log('Button clicked!');
}, []);
return (
<button onClick={handleClick}>
Click Me
</button>
);
}
7. 事件委托
React 内部使用了事件委托机制,将所有事件处理函数委托到文档根节点上。这意味着你不需要手动管理事件委托,React 会自动处理。
8. 自定义事件
虽然 React 提供了丰富的事件类型,但有时你可能需要处理自定义事件。你可以通过 addEventListener
和 removeEventListener
来手动管理自定义事件。
import React, { useEffect } from 'react';
function MyComponent() {
useEffect(() => {
const handleCustomEvent = (event) => {
console.log('Custom event triggered:', event.detail);
};
window.addEventListener('customEvent', handleCustomEvent);
return () => {
window.removeEventListener('customEvent', handleCustomEvent);
};
}, []);
return <div>My Component</div>;
}
总结
React 的事件绑定机制既强大又灵活,能够满足大多数前端开发需求。通过合理使用事件处理函数、传递参数、优化性能等技巧,你可以构建出高效且易于维护的 React 应用。