应用上线后通知用户刷新页面的方法与实践
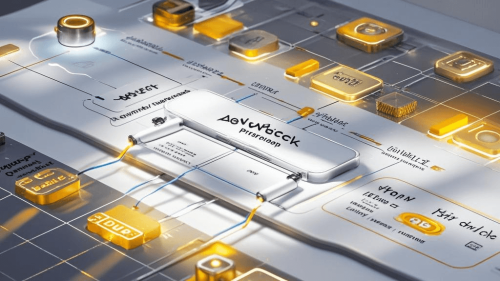
在应用上线后,通知用户刷新当前页面是一个常见的需求,尤其是在应用更新了重要功能或修复了关键问题后。以下是一些常见的实现方式:
1. WebSocket 或 Server-Sent Events (SSE)
- WebSocket: 通过 WebSocket 建立持久连接,服务器可以主动向客户端推送消息,通知用户刷新页面。
- SSE: 类似于 WebSocket,但 SSE 是单向的,服务器可以向客户端推送消息,适合简单的通知场景。
2. 轮询(Polling)
- 定时轮询: 客户端定时向服务器发送请求,检查是否有新版本或更新通知。如果有更新,提示用户刷新页面。
- 长轮询: 客户端发送请求后,服务器保持连接直到有新消息或超时,然后客户端再次发起请求。
3. Service Worker
- Service Worker: 使用 Service Worker 监听应用更新,当检测到新版本时,可以显示提示框或通知,提示用户刷新页面。
- 示例代码:
self.addEventListener('message', (event) => { if (event.data === 'skipWaiting') { self.skipWaiting(); } }); self.addEventListener('controllerchange', () => { window.location.reload(); });
4. 版本控制
- 版本号: 在应用的 HTML 或 JavaScript 文件中嵌入版本号,每次更新时更新版本号。客户端可以定时检查版本号,如果发现版本号变化,提示用户刷新页面。
- 示例代码:
const currentVersion = '1.0.0'; setInterval(async () => { const response = await fetch('/version'); const { version } = await response.json(); if (version !== currentVersion) { alert('新版本已发布,请刷新页面以获取最新内容。'); } }, 60000); // 每分钟检查一次
5. 浏览器通知
- Notification API: 使用浏览器的 Notification API 显示通知,提示用户刷新页面。
- 示例代码:
if (Notification.permission === 'granted') { new Notification('新版本已发布', { body: '请刷新页面以获取最新内容。', }); } else if (Notification.permission !== 'denied') { Notification.requestPermission().then(permission => { if (permission === 'granted') { new Notification('新版本已发布', { body: '请刷新页面以获取最新内容。', }); } }); }
6. UI 提示
- Toast 或 Modal: 在页面顶部或底部显示一个非阻塞的提示框(Toast)或模态框(Modal),提示用户刷新页面。
- 示例代码:
function showRefreshPrompt() { const toast = document.createElement('div'); toast.style.position = 'fixed'; toast.style.bottom = '20px'; toast.style.right = '20px'; toast.style.padding = '10px'; toast.style.backgroundColor = '#333'; toast.style.color = '#fff'; toast.style.borderRadius = '5px'; toast.innerText = '新版本已发布,请刷新页面。'; document.body.appendChild(toast); const closeButton = document.createElement('button'); closeButton.innerText = '×'; closeButton.style.marginLeft = '10px'; closeButton.style.backgroundColor = 'transparent'; closeButton.style.border = 'none'; closeButton.style.color = '#fff'; closeButton.style.cursor = 'pointer'; closeButton.onclick = () => document.body.removeChild(toast); toast.appendChild(closeButton); }
7. 自动刷新
- 自动刷新: 在检测到新版本后,自动刷新页面。这种方式可能会影响用户体验,因此需要谨慎使用。
- 示例代码:
window.location.reload();
8. 混合策略
- 混合策略: 结合多种方式,例如使用 Service Worker 检测更新,然后通过 UI 提示或浏览器通知用户刷新页面。
最佳实践
- 用户体验: 提示用户刷新页面时,尽量使用非阻塞的方式(如 Toast 或 Notification),避免打断用户操作。
- 频率控制: 避免频繁提示用户刷新页面,尤其是在用户正在进行重要操作时。
- 版本管理: 确保版本管理清晰,避免因版本混乱导致用户频繁刷新页面。
通过以上方式,你可以有效地通知用户刷新页面,确保他们使用的是最新版本的应用。