链表的数据结构介绍及应用分析
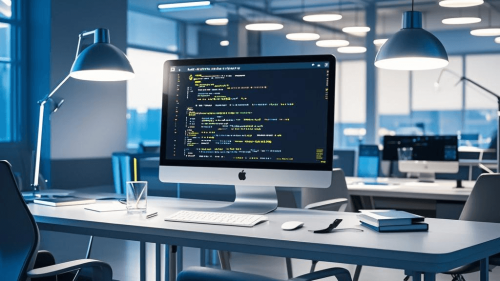
链表(Linked List)是一种常见的数据结构,它由一系列节点(Node)组成,每个节点包含数据和指向下一个节点的指针。与数组不同,链表在内存中是非连续存储的,因此它具有动态分配内存的优势,能够高效地进行插入和删除操作。
1. 链表的类型
- 单向链表(Singly Linked List):每个节点只包含一个指向下一个节点的指针。
- 双向链表(Doubly Linked List):每个节点包含两个指针,分别指向前一个节点和后一个节点。
- 循环链表(Circular Linked List):尾节点的指针指向头节点,形成一个环。
2. 常见的链表操作
-
插入:
- 在链表头部插入:O(1)
- 在链表尾部插入:O(n)(单向链表),O(1)(双向链表)
- 在指定位置插入:O(n)
-
删除:
- 删除链表头部节点:O(1)
- 删除链表尾部节点:O(n)(单向链表),O(1)(双向链表)
- 删除指定位置的节点:O(n)
-
查找:
- 按值查找:O(n)
- 按索引查找:O(n)
-
遍历:O(n)
3. 链表的应用场景
- 动态内存分配:链表适合在需要频繁插入和删除的场景中使用,因为它的内存分配是动态的。
- 实现栈和队列:链表可以用来实现栈(LIFO)和队列(FIFO)数据结构。
- LRU缓存:链表常用于实现LRU(Least Recently Used)缓存淘汰算法。
- 图的邻接表表示:链表可以用来表示图的邻接表,适合稀疏图。
- 多项式表示:链表可以用来表示多项式,每个节点存储一个项的系数和指数。
4. 链表的优缺点
-
优点:
- 动态内存分配,不需要预先知道数据大小。
- 插入和删除操作高效,尤其是双向链表。
-
缺点:
- 访问元素的时间复杂度较高,需要从头节点开始遍历。
- 需要额外的内存空间存储指针。
5. 链表的实现(JavaScript示例)
class Node {
constructor(data) {
this.data = data;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
}
// 在链表头部插入节点
insertAtHead(data) {
const newNode = new Node(data);
newNode.next = this.head;
this.head = newNode;
}
// 在链表尾部插入节点
insertAtTail(data) {
const newNode = new Node(data);
if (!this.head) {
this.head = newNode;
return;
}
let current = this.head;
while (current.next) {
current = current.next;
}
current.next = newNode;
}
// 删除链表头部节点
deleteAtHead() {
if (!this.head) return;
this.head = this.head.next;
}
// 查找节点
find(data) {
let current = this.head;
while (current) {
if (current.data === data) return current;
current = current.next;
}
return null;
}
// 遍历链表
print() {
let current = this.head;
while (current) {
console.log(current.data);
current = current.next;
}
}
}
// 使用示例
const list = new LinkedList();
list.insertAtHead(3);
list.insertAtHead(2);
list.insertAtHead(1);
list.insertAtTail(4);
list.print(); // 输出: 1 2 3 4
list.deleteAtHead();
list.print(); // 输出: 2 3 4
6. 总结
链表是一种灵活的数据结构,适合在需要频繁插入和删除的场景中使用。尽管它的访问效率不如数组,但在某些特定场景下(如动态内存分配、实现栈和队列等),链表是非常有用的工具。理解链表的基本操作和应用场景,对于掌握数据结构和算法至关重要。