常见的JavaScript数据结构介绍
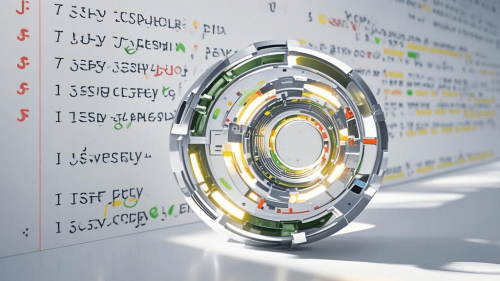
在JavaScript中,数据结构是组织和存储数据的方式,以便有效地访问和修改数据。以下是一些常见的JavaScript数据结构:
-
数组(Array):
- 数组是一种有序的集合,可以存储多个值。数组中的每个值称为元素,可以通过索引访问。
- 示例:
let fruits = ['Apple', 'Banana', 'Cherry']; console.log(fruits[0]); // 输出: Apple
-
对象(Object):
- 对象是无序的键值对集合。键是字符串或符号,值可以是任何数据类型。
- 示例:
let person = { name: 'John', age: 30, isStudent: false }; console.log(person.name); // 输出: John
-
集合(Set):
- 集合是一种无序且唯一的数据结构,不允许重复值。
- 示例:
let uniqueNumbers = new Set([1, 2, 3, 4, 4, 5]); console.log(uniqueNumbers); // 输出: Set { 1, 2, 3, 4, 5 }
-
映射(Map):
- 映射是一种键值对的集合,键可以是任何数据类型。
- 示例:
let map = new Map(); map.set('name', 'Alice'); map.set(1, 'number one'); console.log(map.get('name')); // 输出: Alice
-
栈(Stack):
- 栈是一种后进先出(LIFO)的数据结构。最后添加的元素最先被移除。
- 示例:
let stack = []; stack.push(1); stack.push(2); console.log(stack.pop()); // 输出: 2
-
队列(Queue):
- 队列是一种先进先出(FIFO)的数据结构。最先添加的元素最先被移除。
- 示例:
let queue = []; queue.push(1); queue.push(2); console.log(queue.shift()); // 输出: 1
-
链表(Linked List):
- 链表是一种线性数据结构,每个元素都是一个节点,节点包含数据和指向下一个节点的指针。
- 示例:
class Node { constructor(data) { this.data = data; this.next = null; } } let head = new Node(1); head.next = new Node(2); console.log(head.data); // 输出: 1 console.log(head.next.data); // 输出: 2
-
树(Tree):
- 树是一种分层的数据结构,由节点组成,每个节点有一个父节点和零个或多个子节点。
- 示例:
class TreeNode { constructor(data) { this.data = data; this.children = []; } } let root = new TreeNode(1); root.children.push(new TreeNode(2)); root.children.push(new TreeNode(3)); console.log(root.data); // 输出: 1 console.log(root.children[0].data); // 输出: 2
-
图(Graph):
- 图是一种由节点(顶点)和边组成的非线性数据结构。图可以是有向的或无向的。
- 示例:
class Graph { constructor() { this.nodes = new Map(); } addNode(node) { this.nodes.set(node, []); } addEdge(node1, node2) { this.nodes.get(node1).push(node2); this.nodes.get(node2).push(node1); } } let graph = new Graph(); graph.addNode('A'); graph.addNode('B'); graph.addEdge('A', 'B'); console.log(graph.nodes.get('A')); // 输出: ['B']
-
堆(Heap):
- 堆是一种特殊的树形数据结构,通常用于实现优先队列。堆可以是最大堆或最小堆。
- 示例:
class MinHeap { constructor() { this.heap = []; } insert(value) { this.heap.push(value); this.bubbleUp(); } bubbleUp() { let index = this.heap.length - 1; while (index > 0) { let element = this.heap[index]; let parentIndex = Math.floor((index - 1) / 2); let parent = this.heap[parentIndex]; if (parent <= element) break; this.heap[index] = parent; this.heap[parentIndex] = element; index = parentIndex; } } } let heap = new MinHeap(); heap.insert(3); heap.insert(1); heap.insert(2); console.log(heap.heap); // 输出: [1, 3, 2]
这些数据结构在JavaScript中非常有用,可以根据具体的应用场景选择合适的数据结构来优化性能和代码的可读性。