JavaScript中Array.prototype.map方法的实现与解析
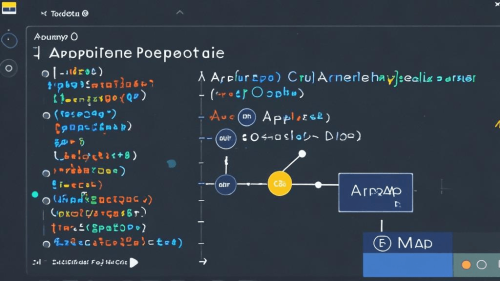
Array.prototype.map
是 JavaScript 中非常常用的数组方法之一,它允许你遍历数组中的每个元素,并对每个元素执行一个回调函数,最终返回一个新的数组,新数组中的每个元素都是回调函数的返回值。
下面是一个简单的实现 map
方法的示例:
Array.prototype.myMap = function(callback, thisArg) {
// 检查 this 是否为 null 或 undefined
if (this == null) {
throw new TypeError('Array.prototype.myMap called on null or undefined');
}
// 检查 callback 是否为函数
if (typeof callback !== 'function') {
throw new TypeError(callback + ' is not a function');
}
// 将 this 转换为对象
const array = Object(this);
// 获取数组的长度
const length = array.length >>> 0;
// 创建一个新数组,用于存储结果
const result = new Array(length);
// 遍历数组
for (let i = 0; i < length; i++) {
// 检查数组中是否存在当前索引
if (i in array) {
// 调用回调函数,并将结果存储在新数组中
result[i] = callback.call(thisArg, array[i], i, array);
}
}
// 返回新数组
return result;
};
// 示例用法
const numbers = [1, 2, 3, 4];
const doubled = numbers.myMap(function(num) {
return num * 2;
});
console.log(doubled); // 输出: [2, 4, 6, 8]
代码解释:
-
参数检查:
this == null
:确保this
不是null
或undefined
。typeof callback !== 'function'
:确保传入的callback
是一个函数。
-
数组长度:
array.length >>> 0
:确保数组长度是一个非负整数。
-
遍历数组:
- 使用
for
循环遍历数组,并通过i in array
检查当前索引是否存在。 - 调用
callback
函数,并将当前元素、索引和原数组作为参数传递给它。
- 使用
-
返回新数组:
- 将回调函数的结果存储在
result
数组中,并最终返回result
。
- 将回调函数的结果存储在
注意事项:
map
方法不会改变原数组,而是返回一个新数组。- 如果数组是稀疏数组(即某些索引没有元素),
map
方法会跳过这些索引。
性能考虑:
map
方法的时间复杂度是 O(n),其中 n 是数组的长度。- 如果你在处理非常大的数组时遇到性能问题,可以考虑使用
for
循环或其他优化手段。
这个实现是一个简单的 map
方法实现,适用于大多数场景。如果你需要更复杂的处理(例如处理异步操作),可能需要进一步扩展这个实现。