React项目中常见的错误捕获方法
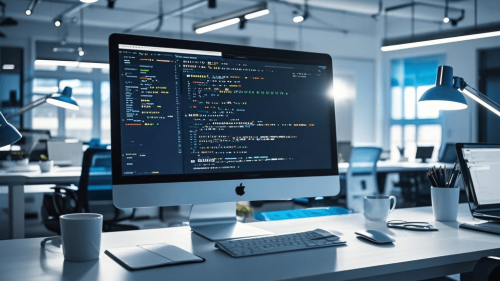
在 React 项目中,捕获错误是确保应用稳定性和用户体验的重要环节。以下是几种常见的捕获错误的方法:
1. Error Boundaries
Error Boundaries 是 React 16 引入的一种机制,用于捕获组件树中的 JavaScript 错误,并显示降级 UI 而不是崩溃的组件树。
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
// 更新 state 使下一次渲染能够显示降级后的 UI
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
// 你同样可以将错误日志上报给服务器
console.error("Caught an error:", error, errorInfo);
}
render() {
if (this.state.hasError) {
// 你可以自定义降级后的 UI 并渲染
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
// 使用 ErrorBoundary 包裹组件
<ErrorBoundary>
<MyComponent />
</ErrorBoundary>
2. try-catch
对于非 React 组件的代码(如事件处理函数、异步操作等),可以使用传统的 try-catch
语句来捕获错误。
function handleClick() {
try {
// 可能会抛出错误的代码
riskyOperation();
} catch (error) {
console.error("Caught an error:", error);
}
}
3. Promise.catch
对于异步操作(如 fetch
请求),可以使用 Promise.catch
来捕获错误。
fetch('/api/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error("Fetch error:", error));
4. 全局错误捕获
可以使用 window.onerror
或 window.addEventListener('error', ...)
来捕获全局的 JavaScript 错误。
window.onerror = function(message, source, lineno, colno, error) {
console.error("Global error:", message, source, lineno, colno, error);
return true; // 阻止默认错误处理
};
window.addEventListener('error', function(event) {
console.error("Global error:", event.error);
});
5. React 生命周期方法中的错误处理
在 React 生命周期方法中,可以使用 try-catch
来捕获错误。
class MyComponent extends React.Component {
componentDidMount() {
try {
// 可能会抛出错误的代码
riskyOperation();
} catch (error) {
console.error("Caught an error:", error);
}
}
render() {
return <div>My Component</div>;
}
}
6. 使用第三方错误监控服务
可以使用如 Sentry、LogRocket 等第三方错误监控服务来捕获和上报错误。
import * as Sentry from '@sentry/react';
import { Integrations } from '@sentry/tracing';
Sentry.init({
dsn: 'YOUR_SENTRY_DSN',
integrations: [new Integrations.BrowserTracing()],
tracesSampleRate: 1.0,
});
// 在 Error Boundary 中使用 Sentry
class ErrorBoundary extends React.Component {
componentDidCatch(error, errorInfo) {
Sentry.captureException(error, { extra: errorInfo });
}
render() {
return this.props.children;
}
}
7. React 18 中的新特性
React 18 引入了 useErrorBoundary
Hook,可以更方便地在函数组件中使用 Error Boundary。
import { useErrorBoundary } from 'react-error-boundary';
function MyComponent() {
const { ErrorBoundary, didCatch, error } = useErrorBoundary();
if (didCatch) {
return <div>Something went wrong: {error.message}</div>;
}
return (
<ErrorBoundary>
<RiskyComponent />
</ErrorBoundary>
);
}
总结
在 React 项目中,捕获错误的方法多种多样,可以根据具体场景选择合适的方式。Error Boundaries 是 React 特有的机制,适合捕获组件树中的错误,而 try-catch
和 Promise.catch
则适用于更广泛的 JavaScript 代码。全局错误捕获和第三方服务则提供了更全面的错误监控和上报能力。