JavaScript中Promise的手动实现与原理
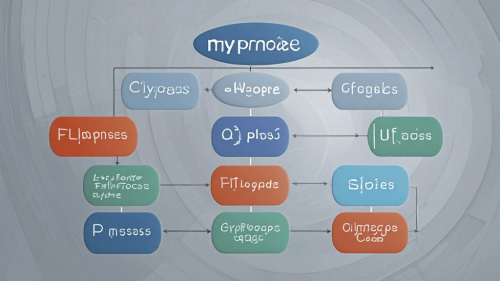
在 JavaScript 中,Promise
是一种用于处理异步操作的对象。它有三种主要的状态:pending
(进行中)、fulfilled
(已成功)和 rejected
(已失败)。Promise
的 resolve
和 reject
方法用于改变 Promise
的状态,而 finally
方法则用于在 Promise
完成(无论成功或失败)后执行一些清理操作。
下面是一个简单的实现,展示了如何手动实现 Promise
的 resolve
、reject
和 finally
方法:
class MyPromise {
constructor(executor) {
this.state = 'pending';
this.value = undefined;
this.reason = undefined;
this.onFulfilledCallbacks = [];
this.onRejectedCallbacks = [];
this.onFinallyCallbacks = [];
const resolve = (value) => {
if (this.state === 'pending') {
this.state = 'fulfilled';
this.value = value;
this.onFulfilledCallbacks.forEach(callback => callback(this.value));
this.onFinallyCallbacks.forEach(callback => callback());
}
};
const reject = (reason) => {
if (this.state === 'pending') {
this.state = 'rejected';
this.reason = reason;
this.onRejectedCallbacks.forEach(callback => callback(this.reason));
this.onFinallyCallbacks.forEach(callback => callback());
}
};
try {
executor(resolve, reject);
} catch (error) {
reject(error);
}
}
then(onFulfilled, onRejected) {
if (this.state === 'fulfilled') {
onFulfilled(this.value);
} else if (this.state === 'rejected') {
onRejected(this.reason);
} else if (this.state === 'pending') {
this.onFulfilledCallbacks.push(onFulfilled);
this.onRejectedCallbacks.push(onRejected);
}
return this;
}
catch(onRejected) {
return this.then(null, onRejected);
}
finally(onFinally) {
if (this.state !== 'pending') {
onFinally();
} else {
this.onFinallyCallbacks.push(onFinally);
}
return this;
}
}
// 使用示例
const promise = new MyPromise((resolve, reject) => {
setTimeout(() => {
resolve('Success!');
// reject('Error!');
}, 1000);
});
promise
.then((value) => {
console.log(value); // 输出: Success!
})
.catch((error) => {
console.error(error); // 输出: Error!
})
.finally(() => {
console.log('Finally!'); // 输出: Finally!
});
解释:
-
resolve
和reject
:resolve
方法将Promise
的状态从pending
改为fulfilled
,并调用所有注册的onFulfilled
回调。reject
方法将Promise
的状态从pending
改为rejected
,并调用所有注册的onRejected
回调。
-
then
方法:then
方法用于注册onFulfilled
和onRejected
回调。如果Promise
已经完成(fulfilled
或rejected
),则立即调用相应的回调;否则,将回调存储在数组中,等待Promise
完成时调用。
-
catch
方法:catch
方法是then(null, onRejected)
的简写,用于处理Promise
的失败情况。
-
finally
方法:finally
方法用于注册一个回调,无论Promise
是成功还是失败,都会执行该回调。它通常用于执行一些清理操作。
注意事项:
- 这个实现是一个简化的版本,实际的
Promise
实现要复杂得多,包括链式调用、微任务队列等。 - 在实际开发中,建议使用原生的
Promise
,而不是自己实现,因为原生的Promise
经过了广泛的测试和优化。
这个实现可以帮助你理解 Promise
的基本工作原理,但在生产环境中,建议使用原生的 Promise
或 async/await
语法。