React 组件创建方式:函数组件与类组件解析
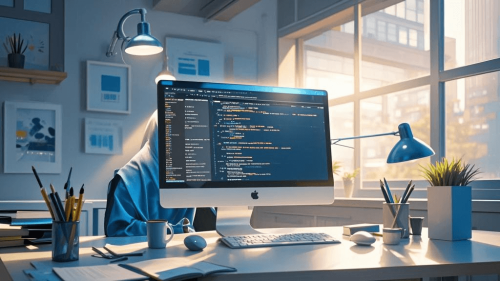
在 React 中,创建组件主要有两种方式:函数组件和类组件。随着 React 16.8 引入 Hooks 后,函数组件的能力得到了极大的增强,现在已经成为主流的组件创建方式。以下是这两种方式的详细介绍:
1. 函数组件(Function Components)
函数组件是使用 JavaScript 函数定义的组件。它们通常更简洁,易于理解和测试。自从 React Hooks 引入后,函数组件也可以使用状态(state)和生命周期方法。
基本语法:
function MyComponent(props) {
return <div>Hello, {props.name}!</div>;
}
使用 Hooks 的函数组件:
import React, { useState, useEffect } from 'react';
function MyComponent(props) {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `You clicked ${count} times`;
}, [count]);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
2. 类组件(Class Components)
类组件是使用 ES6 类定义的组件。它们可以包含状态(state)和生命周期方法。虽然类组件仍然有效,但在现代 React 开发中,函数组件结合 Hooks 更为推荐。
基本语法:
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
componentDidMount() {
document.title = `You clicked ${this.state.count} times`;
}
componentDidUpdate() {
document.title = `You clicked ${this.state.count} times`;
}
render() {
return (
<div>
<p>You clicked {this.state.count} times</p>
<button onClick={() => this.setState({ count: this.state.count + 1 })}>
Click me
</button>
</div>
);
}
}
3. 选择哪种方式?
- 函数组件:推荐使用,尤其是结合 Hooks 后,函数组件可以处理几乎所有类组件的功能,并且代码更简洁、易于维护。
- 类组件:在一些遗留代码库中可能仍然存在,或者在某些特定场景下(如需要继承时)可能会用到。
4. 最佳实践
- 优先使用函数组件:除非有特殊需求,否则优先使用函数组件和 Hooks。
- 保持组件简洁:尽量将组件拆分为更小的、可复用的组件。
- 使用 PropTypes 或 TypeScript:为组件的 props 添加类型检查,提高代码的健壮性。
5. 示例:函数组件与类组件的对比
函数组件:
import React, { useState, useEffect } from 'react';
function Counter() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `Count: ${count}`;
}, [count]);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
类组件:
import React, { Component } from 'react';
class Counter extends Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
componentDidMount() {
document.title = `Count: ${this.state.count}`;
}
componentDidUpdate() {
document.title = `Count: ${this.state.count}`;
}
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={() => this.setState({ count: this.state.count + 1 })}>
Increment
</button>
</div>
);
}
}
总结
在现代 React 开发中,函数组件结合 Hooks 是创建组件的首选方式。它们提供了更简洁的语法和更强大的功能,同时保持了代码的可读性和可维护性。类组件仍然有效,但在大多数情况下,函数组件已经足够应对各种需求。