JavaScript 核心 API 和模式的实现解析
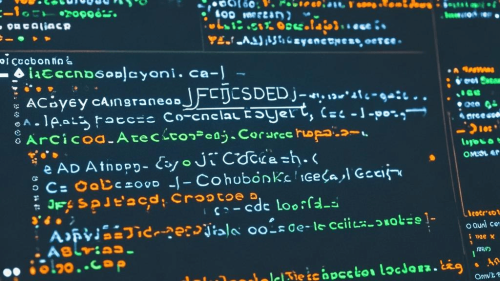
在 JavaScript 中,理解和实现一些核心的 API 和模式对于深入掌握语言特性和提升开发能力至关重要。以下是一些重要的 API 和模式的实现细节:
1. 数组方法
JavaScript 提供了许多内置的数组方法,如 map
, filter
, reduce
等。这些方法可以通过原生 JavaScript 实现。
Array.prototype.myMap = function(callback) {
const result = [];
for (let i = 0; i < this.length; i++) {
result.push(callback(this[i], i, this));
}
return result;
};
Array.prototype.myFilter = function(callback) {
const result = [];
for (let i = 0; i < this.length; i++) {
if (callback(this[i], i, this)) {
result.push(this[i]);
}
}
return result;
};
Array.prototype.myReduce = function(callback, initialValue) {
let accumulator = initialValue === undefined ? this[0] : initialValue;
for (let i = initialValue === undefined ? 1 : 0; i < this.length; i++) {
accumulator = callback(accumulator, this[i], i, this);
}
return accumulator;
};
2. call
和 apply
call
和 apply
用于改变函数的上下文(this
的指向)。
Function.prototype.myCall = function(context, ...args) {
context = context || window;
context.fn = this;
const result = context.fn(...args);
delete context.fn;
return result;
};
Function.prototype.myApply = function(context, args) {
context = context || window;
context.fn = this;
const result = context.fn(...args);
delete context.fn;
return result;
};
3. Object.create
Object.create
用于创建一个新对象,使用现有的对象作为新对象的原型。
Object.myCreate = function(proto) {
function F() {}
F.prototype = proto;
return new F();
};
4. bind
bind
方法创建一个新的函数,在调用时设置 this
关键字为提供的值。
Function.prototype.myBind = function(context, ...args) {
const self = this;
return function(...innerArgs) {
return self.apply(context, args.concat(innerArgs));
};
};
5. new
操作符
new
操作符用于创建一个用户定义的对象类型的实例或具有构造函数的内置对象类型的实例。
function myNew(constructor, ...args) {
const obj = Object.create(constructor.prototype);
const result = constructor.apply(obj, args);
return result instanceof Object ? result : obj;
}
6. instanceof
instanceof
运算符用于检测构造函数的 prototype
属性是否出现在某个实例对象的原型链上。
function myInstanceof(instance, constructor) {
let proto = Object.getPrototypeOf(instance);
while (proto) {
if (proto === constructor.prototype) return true;
proto = Object.getPrototypeOf(proto);
}
return false;
}
7. 单例模式
单例模式确保一个类只有一个实例,并提供一个全局访问点。
const Singleton = (function() {
let instance;
function createInstance() {
const object = new Object("I am the instance");
return object;
}
return {
getInstance: function() {
if (!instance) {
instance = createInstance();
}
return instance;
}
};
})();
8. 防抖(Debounce)
防抖函数用于限制某个函数在短时间内多次触发时只执行一次。
function debounce(func, wait) {
let timeout;
return function(...args) {
clearTimeout(timeout);
timeout = setTimeout(() => func.apply(this, args), wait);
};
}
9. 节流(Throttle)
节流函数用于限制某个函数在一定时间内只执行一次。
function throttle(func, wait) {
let lastTime = 0;
return function(...args) {
const now = Date.now();
if (now - lastTime >= wait) {
func.apply(this, args);
lastTime = now;
}
};
}
10. 深拷贝(Deep Clone)
深拷贝用于创建一个对象的完全独立副本,包括嵌套对象。
function deepClone(obj) {
if (obj === null || typeof obj !== 'object') return obj;
const clone = Array.isArray(obj) ? [] : {};
for (let key in obj) {
if (obj.hasOwnProperty(key)) {
clone[key] = deepClone(obj[key]);
}
}
return clone;
}
11. Promise
Promise
用于处理异步操作,表示一个最终会完成(或失败)的操作及其结果值。
class MyPromise {
constructor(executor) {
this.state = 'pending';
this.value = undefined;
this.reason = undefined;
this.onFulfilledCallbacks = [];
this.onRejectedCallbacks = [];
const resolve = (value) => {
if (this.state === 'pending') {
this.state = 'fulfilled';
this.value = value;
this.onFulfilledCallbacks.forEach(fn => fn());
}
};
const reject = (reason) => {
if (this.state === 'pending') {
this.state = 'rejected';
this.reason = reason;
this.onRejectedCallbacks.forEach(fn => fn());
}
};
try {
executor(resolve, reject);
} catch (error) {
reject(error);
}
}
then(onFulfilled, onRejected) {
if (this.state === 'fulfilled') {
onFulfilled(this.value);
} else if (this.state === 'rejected') {
onRejected(this.reason);
} else if (this.state === 'pending') {
this.onFulfilledCallbacks.push(() => onFulfilled(this.value));
this.onRejectedCallbacks.push(() => onRejected(this.reason));
}
}
}
这些实现展示了 JavaScript 中一些核心概念和模式的底层原理,理解这些实现有助于更好地掌握 JavaScript 的高级特性。