JavaScript中bind、call和apply方法的区别与实现
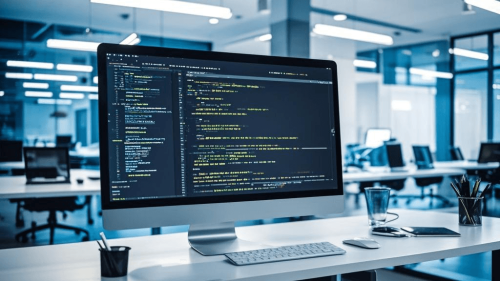
bind
、call
和 apply
是 JavaScript 中用于改变函数执行时 this
指向的三个方法。它们的主要区别在于调用方式和参数传递方式。
1. call
和 apply
的区别
call
:立即执行函数,第一个参数是this
的指向,后续参数是传递给函数的参数列表。apply
:立即执行函数,第一个参数是this
的指向,第二个参数是一个数组(或类数组对象),数组中的元素是传递给函数的参数。
示例:
function greet(greeting, punctuation) {
console.log(greeting + ', ' + this.name + punctuation);
}
const person = { name: 'Alice' };
// 使用 call
greet.call(person, 'Hello', '!'); // 输出: Hello, Alice!
// 使用 apply
greet.apply(person, ['Hi', '!!']); // 输出: Hi, Alice!!
2. bind
的区别
bind
:不会立即执行函数,而是返回一个新的函数,新函数的this
被永久绑定到bind
的第一个参数。后续参数会作为新函数的预设参数。
示例:
function greet(greeting, punctuation) {
console.log(greeting + ', ' + this.name + punctuation);
}
const person = { name: 'Alice' };
// 使用 bind
const boundGreet = greet.bind(person, 'Hello');
boundGreet('!'); // 输出: Hello, Alice!
3. bind
的实现
bind
的实现可以通过以下步骤来完成:
- 返回一个新的函数。
- 在新函数内部通过
call
或apply
来调用原函数,并设置this
和参数。
实现代码:
Function.prototype.myBind = function(context, ...args) {
const self = this; // 保存原函数
return function(...innerArgs) {
// 合并预设参数和新传入的参数
const finalArgs = args.concat(innerArgs);
// 使用 call 或 apply 调用原函数
return self.apply(context, finalArgs);
};
};
// 测试
function greet(greeting, punctuation) {
console.log(greeting + ', ' + this.name + punctuation);
}
const person = { name: 'Alice' };
const boundGreet = greet.myBind(person, 'Hello');
boundGreet('!'); // 输出: Hello, Alice!
总结
call
和apply
:立即执行函数,区别在于参数传递方式。bind
:返回一个新函数,this
被永久绑定,适合用于延迟执行或事件处理函数。bind
的实现:通过闭包和apply
或call
来实现this
的绑定和参数传递。
理解这些方法的区别和实现原理,有助于在实际开发中更好地控制函数的执行上下文和参数传递。