JavaScript数组扁平化方法全解析
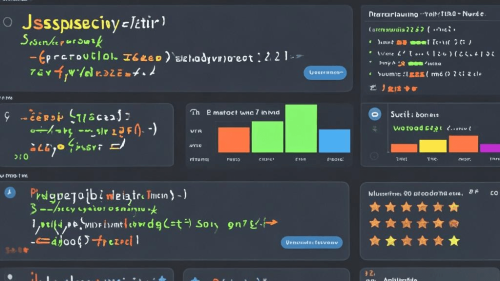
在 JavaScript 中,数组扁平化是指将多维数组转换为一维数组的过程。以下是几种常见的数组扁平化方法:
1. 使用 Array.prototype.flat()
方法
flat()
是 ES2019 引入的方法,可以轻松地将多维数组扁平化。你可以指定扁平化的深度,默认是 1。
const arr = [1, [2, [3, [4]]]];
const flattened = arr.flat(Infinity); // 使用 Infinity 可以扁平化任意深度的嵌套数组
console.log(flattened); // 输出: [1, 2, 3, 4]
2. 使用 Array.prototype.reduce()
和递归
如果你需要在不支持 flat()
的环境中使用,可以使用 reduce()
和递归来实现扁平化。
function flattenArray(arr) {
return arr.reduce((acc, val) => Array.isArray(val) ? acc.concat(flattenArray(val)) : acc.concat(val), []);
}
const arr = [1, [2, [3, [4]]];
const flattened = flattenArray(arr);
console.log(flattened); // 输出: [1, 2, 3, 4]
3. 使用 Array.prototype.concat()
和 apply()
这种方法利用了 concat()
和 apply()
的特性来实现扁平化。
function flattenArray(arr) {
return [].concat.apply([], arr.map(item => Array.isArray(item) ? flattenArray(item) : item));
}
const arr = [1, [2, [3, [4]]];
const flattened = flattenArray(arr);
console.log(flattened); // 输出: [1, 2, 3, 4]
4. 使用 Array.prototype.flatMap()
flatMap()
是 map()
和 flat()
的结合体,但它只能扁平化一层。
const arr = [1, [2, [3, [4]]];
const flattened = arr.flatMap(item => item);
console.log(flattened); // 输出: [1, 2, [3, [4]]]
5. 使用 lodash
库
如果你已经在项目中使用了 lodash
,可以使用 _.flattenDeep()
方法来实现深度扁平化。
const _ = require('lodash');
const arr = [1, [2, [3, [4]]];
const flattened = _.flattenDeep(arr);
console.log(flattened); // 输出: [1, 2, 3, 4]
6. 使用 Generator
函数
你也可以使用 Generator
函数来实现扁平化。
function* flatten(array) {
for (const item of array) {
if (Array.isArray(item)) {
yield* flatten(item);
} else {
yield item;
}
}
}
const arr = [1, [2, [3, [4]]];
const flattened = [...flatten(arr)];
console.log(flattened); // 输出: [1, 2, 3, 4]
总结
flat()
是最简单和推荐的方法,尤其是在现代 JavaScript 环境中。reduce()
和递归 是一种通用的方法,适用于所有环境。lodash
提供了方便的flattenDeep()
方法,适合已经在使用lodash
的项目。Generator
函数 提供了一种函数式编程的方式来处理扁平化。
根据你的项目需求和环境选择合适的扁平化方法。