跨域请求携带其他域名Cookie的方法
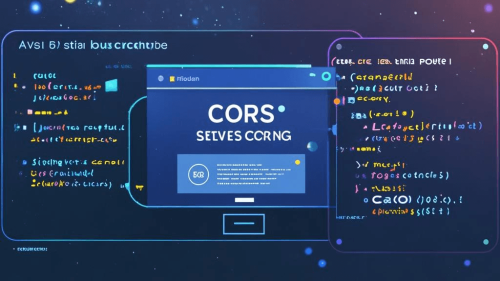
在跨域请求中携带另外一个域名下的 Cookie,需要满足以下几个条件,并按照以下步骤操作:
1. 服务器端设置 CORS 配置
服务器需要在响应头中设置 Access-Control-Allow-Origin
和 Access-Control-Allow-Credentials
。
Access-Control-Allow-Origin
:不能设置为*
,必须明确指定允许的域名。Access-Control-Allow-Credentials
:必须设置为true
。
例如,在 Node.js 中使用 Express 框架时,可以这样配置:
const express = require('express');
const app = express();
app.use((req, res, next) => {
res.header('Access-Control-Allow-Origin', 'https://example.com'); // 允许的域名
res.header('Access-Control-Allow-Credentials', 'true'); // 允许携带凭证
next();
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
2. 客户端设置 withCredentials
在客户端发起请求时,需要将 withCredentials
设置为 true
,以允许跨域请求携带 Cookie。
- 使用原生
XMLHttpRequest
:
const xhr = new XMLHttpRequest();
xhr.open('GET', 'https://api.example.com/data', true);
xhr.withCredentials = true; // 允许携带 Cookie
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
console.log(xhr.responseText);
}
};
xhr.send();
- 使用
fetch
API:
fetch('https://api.example.com/data', {
credentials: 'include' // 允许携带 Cookie
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
- 使用 Axios:
axios.get('https://api.example.com/data', {
withCredentials: true // 允许携带 Cookie
})
.then(response => console.log(response.data))
.catch(error => console.error('Error:', error));
3. Cookie 的 SameSite 属性
确保 Cookie 的 SameSite
属性设置为 None
,并且标记为 Secure
(即仅通过 HTTPS 传输)。
例如,在服务器端设置 Cookie 时:
res.cookie('session', '12345', {
httpOnly: true,
secure: true, // 仅通过 HTTPS 传输
sameSite: 'none' // 允许跨站请求携带 Cookie
});
4. 浏览器兼容性
确保浏览器支持跨域携带 Cookie 的行为。现代浏览器(如 Chrome、Firefox、Edge 等)都支持这一特性,但旧版浏览器可能会有兼容性问题。
总结
要在跨域请求中携带另外一个域名下的 Cookie,需要:
- 服务器端正确配置 CORS 响应头。
- 客户端在请求中设置
withCredentials
为true
。 - 确保 Cookie 的
SameSite
属性为None
并且标记为Secure
。
通过这些步骤,你可以成功在跨域请求中携带另外一个域名下的 Cookie。