Node.js实现代码行数统计命令行工具
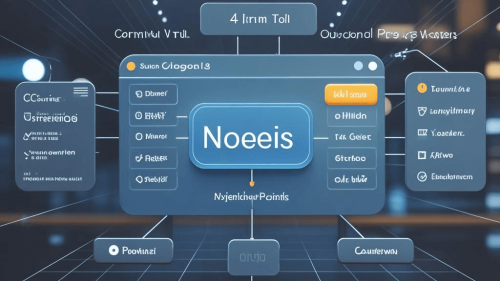
要实现一个用 Node.js 编写的命令行工具来统计指定目录下特定代码文件的行数,你可以按照以下步骤进行。这个工具将允许用户指定目录和文件扩展名,然后统计这些文件的总行数。
1. 创建项目
首先,创建一个新的 Node.js 项目:
mkdir code-line-counter
cd code-line-counter
npm init -y
2. 安装依赖
我们将使用 commander
来处理命令行参数,使用 glob
来匹配文件模式:
npm install commander glob
3. 创建主脚本文件
在项目根目录下创建一个 index.js
文件:
#!/usr/bin/env node
const fs = require('fs');
const path = require('path');
const { program } = require('commander');
const glob = require('glob');
// 定义命令行参数
program
.version('1.0.0')
.description('A CLI tool to count lines of code in a directory')
.requiredOption('-d, --directory <dir>', 'Directory to search')
.requiredOption('-e, --extension <ext>', 'File extension to count (e.g., .js, .ts)')
.parse(process.argv);
const options = program.opts();
// 统计指定目录下特定扩展名文件的行数
function countLinesInDirectory(directory, extension) {
const pattern = path.join(directory, `**/*${extension}`);
let totalLines = 0;
glob(pattern, (err, files) => {
if (err) {
console.error('Error finding files:', err);
return;
}
files.forEach(file => {
const content = fs.readFileSync(file, 'utf8');
const lines = content.split('\n').length;
totalLines += lines;
console.log(`${file}: ${lines} lines`);
});
console.log(`\nTotal lines in ${extension} files: ${totalLines}`);
});
}
// 执行统计
countLinesInDirectory(options.directory, options.extension);
4. 添加可执行权限
为了让这个脚本可以直接从命令行运行,你需要给它添加可执行权限,并在 package.json
中添加 bin
字段:
chmod +x index.js
然后在 package.json
中添加:
{
"name": "code-line-counter",
"version": "1.0.0",
"description": "A CLI tool to count lines of code in a directory",
"main": "index.js",
"bin": {
"code-line-counter": "./index.js"
},
"scripts": {
"start": "node index.js"
},
"dependencies": {
"commander": "^9.0.0",
"glob": "^7.2.0"
}
}
5. 全局安装
你可以将这个工具全局安装,以便在任何地方使用:
npm install -g .
6. 使用工具
现在你可以使用这个工具来统计指定目录下特定扩展名文件的行数了。例如:
code-line-counter -d ./src -e .js
这将统计 ./src
目录下所有 .js
文件的总行数。
7. 进一步优化
- 异步处理:为了提高性能,可以使用异步文件读取和行数统计。
- 忽略特定文件或目录:可以通过
.gitignore
或自定义忽略规则来排除某些文件或目录。 - 支持多种扩展名:可以扩展工具以支持同时统计多种文件扩展名的行数。
这个工具是一个简单的示例,展示了如何使用 Node.js 创建一个命令行工具来统计代码行数。你可以根据实际需求进一步扩展和优化它。