TypeScript常见高级类型全解析
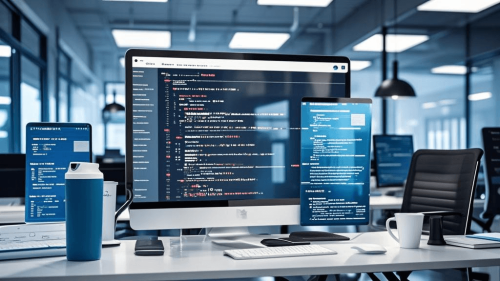
TypeScript 提供了多种高级类型,这些类型可以帮助开发者更精确地定义和操作类型。以下是一些常见的高级类型:
-
联合类型(Union Types):
允许一个变量可以是多种类型中的一种。例如:let value: string | number; value = "Hello"; // 合法 value = 42; // 合法
-
交叉类型(Intersection Types):
将多个类型合并为一个类型。例如:type A = { a: number }; type B = { b: string }; type C = A & B; let obj: C = { a: 1, b: "hello" };
-
类型别名(Type Aliases):
为类型创建一个新名字。例如:type StringOrNumber = string | number; let value: StringOrNumber;
-
索引类型(Index Types):
通过索引访问类型的属性。例如:function getProperty<T, K extends keyof T>(obj: T, key: K) { return obj[key]; } let obj = { a: 1, b: "hello" }; let a = getProperty(obj, "a"); // a 的类型是 number
-
映射类型(Mapped Types):
基于旧类型创建新类型。例如:type Readonly<T> = { readonly [P in keyof T]: T[P]; }; type Person = { name: string; age: number }; type ReadonlyPerson = Readonly<Person>;
-
条件类型(Conditional Types):
根据条件选择类型。例如:type NonNullable<T> = T extends null | undefined ? never : T; type T1 = NonNullable<string | number | null>; // T1 的类型是 string | number
-
类型推断(Type Inference):
自动推断变量的类型。例如:let x = 3; // x 的类型被推断为 number
-
类型保护(Type Guards):
在运行时检查类型。例如:function isString(test: any): test is string { return typeof test === "string"; } if (isString(value)) { console.log(value.toUpperCase()); }
-
类型断言(Type Assertions):
手动指定变量的类型。例如:let someValue: any = "this is a string"; let strLength: number = (someValue as string).length;
-
模板字面量类型(Template Literal Types):
基于字符串字面量创建类型。例如:type World = "world"; type Greeting = `hello ${World}`; // Greeting 的类型是 "hello world"
这些高级类型使得 TypeScript 在类型系统中更加灵活和强大,能够处理更复杂的类型场景。